Can you concatenate arrays in JavaScript?
Table of Contents
Can you concatenate arrays in JavaScript?
concat() The concat() method is used to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array.
How do you combine array arrays?
1. Immutable merge of arrays
- 1.1 Merge using the spread operator. If you want to know one but a good way to merge arrays in JavaScript, then remember the merge using the spread operator.
- 1.2 Merge using array.concat() method.
How do I merge an array of objects into one?

How to merge array objects into one object in JavaScript
- array. concat() to merge objects into one object.
- array. Map() to merge objects into one object.
- reduce() to merge objects into one object.
- Spread operator to merge objects into one object.
How do you combine two elements of arrays in Java?
In order to merge two arrays, we find its length and stored in fal and sal variable respectively. After that, we create a new integer array result which stores the sum of length of both arrays. Now, copy each elements of both arrays to the result array by using arraycopy() function.
How do I merge two arrays in node JS?
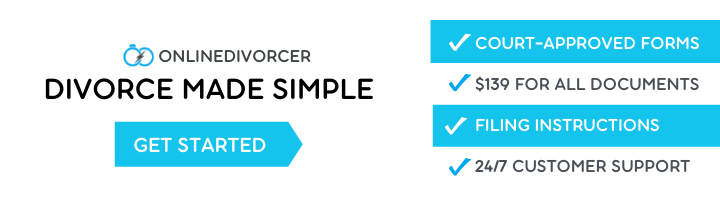
The concat() method is used to merge two or more arrays and is built directly into the Node. js language. It doesn’t change anything about the existing arrays and just simply combines them into one new array.
How do you merge objects in JavaScript?
The easiest way to merge two objects in JavaScript is with the ES6 spread syntax / operator ( ). All you have to do is insert one object into another object along with the spread syntax and any object you use the spread syntax on will be merged into the parent object.
How do you merge two arrays of objects in JavaScript and de duplicate items?
concat() can be used to merge multiple arrays together. But, it does not remove duplicates. filter() is used to identify if an item is present in the “merged_array” or not. Just like the traditional for loop, filter uses the indexOf() method to judge the occurrence of an item in the merged_array.
How do I combine two unique values in an array?
Then, to use it: var array1 = [“Vijendra”,”Singh”]; var array2 = [“Singh”, “Shakya”]; // Merges both arrays and gets unique items var array3 = array1. concat(array2). unique();
How do I merge two arrays of objects in TypeScript?
TypeScript – Array concat() concat() method returns a new array comprised of this array joined with two or more arrays.
What happens if we add two arrays?
In summary, the addition of arrays causes them to be coerced into strings, which does that by joining the elements of the arrays in a comma-separated string and then string-concatenating the joined strings.
How merge two arrays and remove duplicates?
5 ways to Merge two Arrays and Remove Duplicates in Javascript
- Merge arrays and de-duplicate items using concat() and filter()
- Merge arrays and de-duplicate items using Set and spread operator.
- Merge arrays and de-duplicate items using Set and concat()
- Merge arrays and de-duplicate items using while loop and concat()
How do you merge arrays without duplicates?
How can I merge properties of two JavaScript objects dynamically?
The two most common ways of doing this are:
- Using the spread operator ( )
- Using the Object. assign() method.