What is the NUL character in Java?
Table of Contents
What is the NUL character in Java?
”
In the Java programming language, unlike C, an array of char is not a String , and neither a String nor an array of char is terminated by ” (the NUL character).
Does Java have NULL char?
There’s no such entity as NULL in Java. There is null , but that is not a valid value for a char variable. A char can have a value of zero, but that has no particular significance.
How do you pass an empty character in Java?
We can use value to create an empty char in Java. The Java compiler uses this value to set as char initial default value. It represents null that shows empty char. See the example below.

How do you get rid of null in Java?
string. replace(“null”, “”); You can also replace all the instances of ‘null’ with empty String using replaceAll.
What is escape sequence for null character?
sequence \0
The null character is often represented as the escape sequence \0 in source code , string literals or character constants.
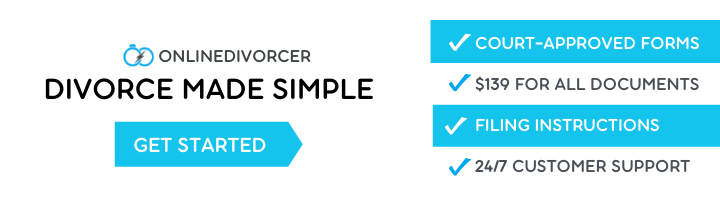
Do strings in Java end with a null?
Java strings are not terminated with a null characters as in C or C++. Although java strings uses internally the char array but there is no terminating null in that. String class provides a method called length to know the number of characters in the string.
How do you replace a character in a string with empty character?
If you call string. Replace(char oldChar, char newChar) it will replace the occurrences of a character with another character. It is a one-for-one replacement. Because of this the length of the resulting string will be the same.
How do I remove a null character from a string?
In short, use the rstrip method for the string. Create a null-terminated string and apply rstrip to it. By default, rstrip will strip away new-line, carriage-return and white space characters — not nulls — which is why I have included the parameter.
How do you remove null from a list?
Algorithm:
- Get the list with null values.
- Create a Stream from the list using list. stream()
- Filter the stream of elements that are not null using list. filter(x -> x != null)
- Collect back the Stream as List using . collect(Collectors. toList()
- Return/Print the list (now with all null values removed).
What are the escape sequences in Java?
Escape Sequences
Escape Sequence | Description |
---|---|
\t | Insert a tab in the text at this point. |
\b | Insert a backspace in the text at this point. |
\n | Insert a newline in the text at this point. |
\r | Insert a carriage return in the text at this point. |
What is terminating NULL character?
All character strings are terminated with a null character. The null character indicates the end of the string. Such strings are called null-terminated strings. The null terminator of a multibyte string consists of one byte whose value is 0.
How do you replace a specific character in a string in Java?
Java String replace(char old, char new) method example
- public class ReplaceExample1{
- public static void main(String args[]){
- String s1=”javatpoint is a very good website”;
- String replaceString=s1.replace(‘a’,’e’);//replaces all occurrences of ‘a’ to ‘e’
- System.out.println(replaceString);
- }}
How do you replace a string after a specific character in Java?
Java String replace() method replaces every occurrence of a given character with a new character and returns a new string. The Java replace() string method allows the replacement of a sequence of character values. The Java replace() function returns a string by replacing oldCh with newCh.
How do I remove a null character from a list in Python?
Let’s discuss certain way outs to remove empty strings from list of strings.
- Method #1 : Using remove()
- Method #2 : Using List Comprehension.
- Method #3 : Using join() + split()
- Method #4 : Using filter()