What is sum product numpy?
Table of Contents
What is sum product numpy?
In the NumPy v1. 15 Reference Guide, the documentation for numpy. dot uses the concept of “sum product”. Namely, we read the following: If a is an N-D array and b is a 1-D array, it is a sum product over the last axis of a and b.
How does numpy calculate sum?
The numpy. sum() function is available in the NumPy package of Python. This function is used to compute the sum of all elements, the sum of each row, and the sum of each column of a given array….Example 2:
- import numpy as np.
- a=np. array([0.4,0.5,0.9,6.1])
- x=np. sum(a, dtype=np. int32)
- x.
How does numpy calculate dot product?
To compute dot product of numpy nd arrays, you can use numpy. dot() function. numpy. dot() functions accepts two numpy arrays as arguments, computes their dot product and returns the result.

How do you sum a numpy matrix?
Use the numpy. sum() Function to Find the Sum of Columns of a Matrix in Python. The sum() function calculates the sum of all elements in an array over the specified axis. If we specify the axis as 0, then it calculates the sum over columns in a matrix.
How do you sum an element of an array in Python?
12. Python program to print the sum of all elements in an array
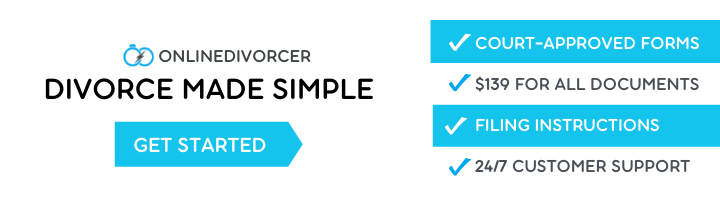
- STEP 1: Declare and initialize an array.
- STEP 2: The variable sum will be used to calculate the sum of the elements. Initialize it to 0.
- STEP 3: Loop through the array and add each element of the array to the variable sum as sum = sum + arr[i].
What is dot () in Python?
dot() in Python. The numpy module of Python provides a function to perform the dot product of two arrays. If both the arrays ‘a’ and ‘b’ are 1-dimensional arrays, the dot() function performs the inner product of vectors (without complex conjugation).
How do you sum in Python?
To find a sum of the List in Python, use the sum() method. The sum() is a built-in method that is used to get the summation. You need to define the List and pass the List as a parameter to the sum() function, and you will get the sum of list items in return.
How do you sum an array in Python?
STEP 1: Declare and initialize an array. STEP 2: The variable sum will be used to calculate the sum of the elements. Initialize it to 0. STEP 3: Loop through the array and add each element of the array to the variable sum as sum = sum + arr[i].
How does numpy multiply matrices?
There are three main ways to perform NumPy matrix multiplication:
- dot(array a, array b) : returns the scalar or dot product of two arrays.
- matmul(array a, array b) : returns the matrix product of two arrays.
- multiply(array a, array b) : returns the element-wise matrix multiplication of two arrays.
How do you sum items in an array?
S = sum( A ) returns the sum of the elements of A along the first array dimension whose size does not equal 1.
- If A is a vector, then sum(A) returns the sum of the elements.
- If A is a matrix, then sum(A) returns a row vector containing the sum of each column.
How do you add the sum of an array?
To find the sum of elements of an array.
- create an empty variable. ( sum)
- Initialize it with 0 in a loop.
- Traverse through each element (or get each element from the user) add each element to sum.
- Print sum.
How do you multiply NumPy arrays?
How do you sum items in a list in Python?
How to compute the sum of a list in python
- def sum_of_list(l): total = 0. for val in l: total = total + val. return total. my_list = [1,3,5,2,4]
- def sum_of_list(l,n): if n == 0: return l[n]; return l[n] + sum_of_list(l,n-1) my_list = [1,3,5,2,4]
- my_list = [1,3,5,2,4] print “The sum of my_list is”, sum(my_list) Run.
How do you sum a series in Python?
Series Summation Using the sum() Function in Python The sum() function sums a list of values in Python. We can use this sum() function with a list comprehension to get the desired list of values for summation. We again have to specify n+1 as the upper limit of the range() function.
Why do we calculate dot product?
The dot product essentially tells us how much of the force vector is applied in the direction of the motion vector. The dot product can also help us measure the angle formed by a pair of vectors and the position of a vector relative to the coordinate axes.
What is the product of 2 vectors?
The Vector product of two vectors, a and b, is denoted by a × b. Its resultant vector is perpendicular to a and b. Vector products are also called cross products. Cross product of two vectors will give the resultant a vector and calculated using the Right-hand Rule.
What is matrix product in NumPy?
Matrix product with numpy. The matmul() function gives us the matrix product of two 2-d arrays. With this method, we can’t use scalar values for our input. If one of our arguments is a 1-d array, the function converts it into a matrix by appending a 1 to its dimension. This is removed after the multiplication is done.
How do you do NumPy element wise multiplication?
The np. multiply(x1, x2) method of the NumPy library of Python takes two matrices x1 and x2 as input, performs element-wise multiplication on input, and returns the resultant matrix as input. Therefore, we need to pass the two matrices as input to the np. multiply() method to perform element-wise input.