What does strncpy mean in C++?
Table of Contents
What does strncpy mean in C++?
C++ strncpy() function The strncpy() function in C++ copies a specified bytes of characters from source to destination.
What does strncpy () do?
The C library function char *strncpy(char *dest, const char *src, size_t n) copies up to n characters from the string pointed to, by src to dest. In a case where the length of src is less than that of n, the remainder of dest will be padded with null bytes.
What is the difference between strncpy and Strncpy_s?
strcpy is a unsafe function. When you try to copy a string using strcpy() to a buffer which is not large enough to contain it, it will cause a buffer overflow. strcpy_s() is a security enhanced version of strcpy() .

What can I use instead of strncpy?
C11 Annex K specifies the strncpy_s() and strncat_s() functions as close replacements for strncpy() and strncat(). The strncpy_s() function copies not more than a specified number of successive characters (characters that follow a null character are not copied) from a source string to a destination character array.
How do you write a strncpy?
Implement strncpy() function in C Write an efficient function to implement strncpy() like function in C, which copies the given n characters from source C-string to another string. The prototype of the strncpy() is: char* strncpy(char* destination, const char* source, size_t num);
Does strncpy allocate memory?
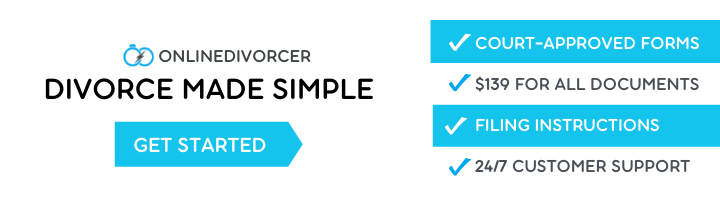
strcpy itself doesn’t allocate memory for the destination string so, no, it doesn’t have to be freed. Of course, if something else had allocated memory for it, then, yes, that memory should be freed eventually but that has nothing to do with strcpy .
How is strncpy implemented?
What is the difference between memcpy and strncpy?
strcpy () is meant for strings only whereas memcpy() is generic function to copy bytes from source to destination location.
What is the difference between strcpy and strncpy?
strcpy( ) function copies whole content of one string into another string. Whereas, strncpy( ) function copies portion of contents of one string into another string. If destination string length is less than source string, entire/specified source string value won’t be copied into destination string in both cases.
Is strncpy thread safe?
strcpy() and strdup() are not thread safe, they are thread agnostic. The only memory locations accessed by those functions are their own local variables, and locations to which your program provides pointers.
Is strncpy safe?
The strncpy() function is insecure because if the NULL character is not available in the first n characters in the source string then the destination string will not be NULL terminated. A program that demonstrates strncpy() in C++ is given as follows.
Why is strncpy insecure?
The strncpy() function is insecure because if the NULL character is not available in the first n characters in the source string then the destination string will not be NULL terminated.
Why memset is used?
memset() is used to fill a block of memory with a particular value.
Is memset faster than for loop?
It depends. But, for sure memset is faster or equal to the for-loop. If you are uncertain of your environment or too lazy to test, take the safe route and go with memset.
What is the difference between Strncpy and memcpy?
Is memset faster than memcpy?
Notice that memcpy is only slightly slower then memset . The operations a[j] += b[j] (where j goes over [0,LEN) ) should take three times longer than memcpy because it operates on three times as much data. However it’s only about 2.5 as slow as memset .