How to sum array in jQuery?
Table of Contents
How to sum array in jQuery?
9 Answers
- (Older) JavaScript: var arr = [“20.0″,”40.1″,”80.2″,”400.3”], n = arr. length, sum = 0; while(n–) sum += parseFloat(arr[n]) || 0;
- ECMA 5.1/6: var arr = [“20.0″,”40.1″,”80.2″,”400.3”], sum = 0; arr.
- ES6: var sum = [“20.0″,”40.1″,”80.2″,”400.3”].
- jQuery: var arr = [“20.0″,”40.1″,”80.2″,”400.3”], sum = 0; $.
How to sum numbers in array JavaScript?
Use the for Loop to Sum an Array in a JavaScript Array Copy const array = [1, 2, 3, 4]; let sum = 0; for (let i = 0; i < array. length; i++) { sum += array[i]; } console. log(sum); We initialize a variable sum as 0 to store the result and use the for loop to visit each element and add them to the sum of the array.
How to get the sum of numbers in JavaScript?
const num1 = parseInt(prompt(‘Enter the first number ‘)); const num2 = parseInt(prompt(‘Enter the second number ‘)); Then, the sum of the numbers is computed. const sum = num1 + num2; Finally, the sum is displayed.

How do you add a large integer to an array?
Approach: The idea is based on adding the digits at corresponding positions of all the numbers. Create an array result[] of size K + 1 to store the result. Traverse all the strings from the end and keep on adding the digits of all the numbers at the same position and insert it into the corresponding index in result[].
Can you do += in JavaScript?
What does += mean in JavaScript? The JavaScript += operator takes the values from the right of the operator and adds it to the variable on the left. This is a very concise method to add two values and assign the result to a variable hence it is called the addition assignment operator.
Why do we use += in JavaScript?
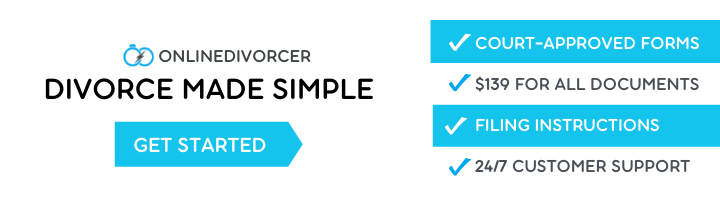
The addition assignment operator ( += ) adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible.
How do you do addition in JavaScript?
Add numbers in JavaScript by placing a plus sign between them. You can also use the following syntax to perform addition: var x+=y; The “+=” operator tells JavaScript to add the variable on the right side of the operator to the variable on the left.
How do you add two numbers together?
When adding, always line up the addends, the two numbers being combined, one on top of each other according to their place values. Add the numbers in the ones column first, then the tens column, and finally the hundreds column, to get the sum, or the total.
How do you add numbers to a linked list?
Traverse the two linked lists in order to add preceding zeros in case a list is having lesser digits than the other one. Start from the head node of both lists and call a recursive function for the next nodes. Continue it till the end of the lists. Creates a node for current digits sum and returns the carry.
How do you store large number of elements in an array?
Use heap memory ( using new keyword) For example if you want to declare a 1000 x 1000 integer array. int **arr = new int*[1000]; for (int i = 0 ; i < 1000 ; i++) arr[i] = new int[1000]; An alternate approach could be to use vector library (vector – C++ Reference). std::vector< std::vector< int > > a;…
How do you input a number in JavaScript?
Input Number value Property
- Change the number of a number field: getElementById(“myNumber”). value = “16”;
- Get the number of a number field: getElementById(“myNumber”). value;
- An example that shows the difference between the defaultValue and value property: getElementById(“myNumber”); var defaultVal = x. defaultValue;
How do you add two elements in a linked list?
Following are the steps.
- Calculate sizes of given two linked lists.
- If sizes are same, then calculate sum using recursion. Hold all nodes in recursion call stack till the rightmost node, calculate the sum of rightmost nodes and forward carry to the left side.
- If size is not same, then follow below steps:
How do I concatenate numbers in an array in JavaScript?
in javascript, you can concat numbers by + or strings as well . I will give you an example : You can look to this. You should convert your array to integers (parseInt) or floats (parseFloat) in this function array_new.reduce ( (a, b) => a + b, 0); It will be something like Thanks for contributing an answer to Stack Overflow!
How to create numbers from strings in JavaScript?
Use parseFloat to create numbers from the strings. in javascript, you can concat numbers by + or strings as well . I will give you an example : You can look to this. You should convert your array to integers (parseInt) or floats (parseFloat) in this function array_new.reduce ( (a, b) => a + b, 0); It will be something like
How to append multiple arrays in JavaScript?
For JavaScript arrays, you use Both push () and concat () function. var array = [1, 2, 3]; array.push (4, 5); //use push for appending a single array. var array1 = [1, 2, 3]; var array2 = [4, 5, 6]; var array3 = array1.concat (array2); //It is better use concat for appending more then one array. Show activity on this post.
How do I convert an array to a number?
Your elements in the array are treated as strings, therefore the + in your reduce function concatenates the array elements into another string. Try to use parseFloat () to treat them as numbers: EDIT: Thanks to charlietfl who mentioned, that parseFloat (a) is redundant, fixed it.