How do you split a string by delimiter in Python?
Table of Contents
How do you split a string by delimiter in Python?
Split strings in Python (delimiter, line break, regex, etc.)
- Split by delimiter: split() Specify the delimiter: sep.
- Split from right by delimiter: rsplit()
- Split by line break: splitlines()
- Split by regex: re.split()
- Concatenate a list of strings.
- Split based on the number of characters: slice.
How do I split a string into multiple strings in Python?
Split String in Python. To split a String in Python with a delimiter, use split() function. split() function splits the string into substrings and returns them as an array.
How do you split a column by delimiter in Python?
Split column by delimiter into multiple columns Apply the pandas series str. split() function on the “Address” column and pass the delimiter (comma in this case) on which you want to split the column. Also, make sure to pass True to the expand parameter.

How do I split text in a column into multiple rows in Python?
To split text in a column into multiple rows with Python Pandas, we can use the str. split method. to create the df data frame.
How do you split letters in Python?
Use list() to split a word into a list of letters
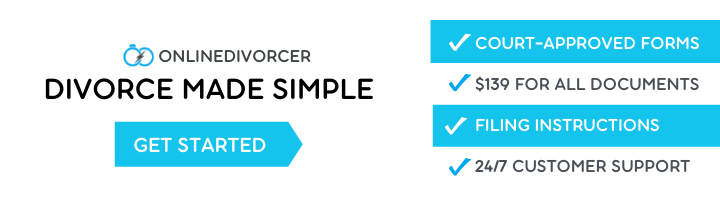
- word = “word”
- list_of_letters = list(word)
- print(list_of_letters)
How do I split text in a column into multiple rows?
The easiest method is to follow these steps:
- Select one cell in your data and press Ctrl+T to convert the data to a table.
- In the Power Query tools, choose From Table.
- Select the column with your products.
- In the Split Column dialog, click on Advanced Options.
- In the Split Into section, choose Rows.
How do you split a string into 3 in Python?
Python 3 – String split() Method
- Description. The split() method returns a list of all the words in the string, using str as the separator (splits on all whitespace if left unspecified), optionally limiting the number of splits to num.
- Syntax.
- Parameters.
- Return Value.
- Example.
- Result.
How do you separate a string from a delimiter?
Example of Pipe Delimited String
- public class SplitStringExample5.
- {
- public static void main(String args[])
- {
- //defining a String object.
- String s = “Life|is|your|creation”;
- //split string delimited by comma.
- String[] stringarray = s.split(“|”); //we can use dot, whitespace, any character.
How do I split text in a column into multiple rows Python?
1 Method-1
- The columns containing multiple values are split, transformed by the stack() method, and accomplished by setting the index.
- Delete columns with multiple values from the DataFrame using the drop() method.
- Then use the join() method to merge.
How do I split a column into multiple rows in Python?
To split text in a column into multiple rows with Python Pandas, we can use the str. split method. to create the df data frame. Then we call str.
How do you split a string into 3 parts?
Solution:
- Get the size of the string using string function strlen() (present in the string.h)
- Get the size of a part. part_size = string_length/n.
- Loop through the input string. In loop, if index becomes multiple of part_size then put a part separator(“\n”)