How do you remove duplicates from an array in C++?
Table of Contents
How do you remove duplicates from an array in C++?
Algorithm to remove duplicate elements in an array (sorted array)
- Input the number of elements of the array.
- Input the array elements.
- Repeat from i = 1 to n.
- – if (arr[i] != arr[i+1])
- – temp[j++] = arr[i]
- – temp[j++] = arr[n-1]
- Repeat from i = 1 to j.
- – arr[i] = temp[i]
How do you remove duplicates from an array of arrays?
To remove duplicates from an array:
- First, convert an array of duplicates to a Set . The new Set will implicitly remove duplicate elements.
- Then, convert the set back to an array.
How do you find the number of duplicates in an array C++?
Find All Duplicates in an Array in C++

- n := size of array, make one array called ans.
- for i in range 0 to n – 1. x := absolute value of nums[i] decrease x by 1. if nums[x] < 0, then add x + 1 into ans, otherwise nums[x] := nums[x] * (-1)
- return ans.
How do you find duplicates in an array?
Algorithm
- Declare and initialize an array.
- Duplicate elements can be found using two loops. The outer loop will iterate through the array from 0 to length of the array. The outer loop will select an element.
- If a match is found which means the duplicate element is found then, display the element.
Does spread operator remove duplicates?
Use the spread operator to remove duplicates items and to sort a array of numbers and objects, without destruct the original array.
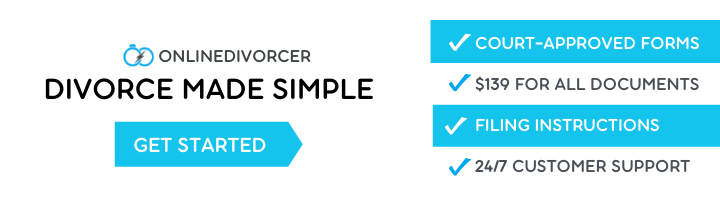
How do you make an array unique?
- Minimum Increment operations to make Array unique.
- Making elements distinct in a sorted array by minimum increments.
- Find sum of non-repeating (distinct) elements in an array.
- Find k closest numbers in an unsorted array.
- Find k closest elements to a given value.
- Search in an almost sorted array.
How do I reindex an array?
The re-index of an array can be done by using some inbuilt function together. These functions are: array_combine() Function: The array_combine() function is an inbuilt function in PHP which is used to combine two arrays and create a new array by using one array for keys and another array for values.
How do you check for duplicates in an array?
One of the most common ways to find duplicates is by using the brute force method, which compares each element of the array to every other element. This solution has the time complexity of O(n^2) and only exists for academic purposes.
How do you count duplicate elements in an array?
To count the duplicates in an array, declare an empty object variable that will store the count for each value and use the forEach() method to iterate over the array. On each iteration, increment the count for the value by 1 or initialize it to 1 if it hasn’t been set already.
Can you do array size () in C++?
array::size() in C++ STL The introduction of array class from C++11 has offered a better alternative for C-style arrays. size() function is used to return the size of the list container or the number of elements in the list container.
How do I get the length of an array of strings in C++?
sizeof(array[0]) will return the size of the pointer corresponding to the first string. Thus, sizeof(array)/sizeof(array[0]) returns the number of strings.
How do you find the length of an array in C++?
How to find the length of an array in C++
- #include
- using namespace std;
-
- int main() {
- int arr[] = {10,20,30,40,50,60};
- int arrSize = sizeof(arr)/sizeof(arr[0]);
- cout << “The size of the array is: ” << arrSize;
- return 0;
How will you sort an array with many duplicated values?
A simple solution would be to use efficient sorting algorithms like Merge Sort, Quicksort, Heapsort, etc., that can solve this problem in O(n. log(n)) time, but those will not take advantage of the fact that there are many duplicated values in the array. A better approach is to use a counting sort.
Does an array have a fixed length?
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed.
How do I remove duplicates from a set?
Approach:
- Take a Set.
- Insert all array element in the Set. Set does not allow duplicates and sets like LinkedHashSet maintains the order of insertion so it will remove duplicates and elements will be printed in the same order in which it is inserted.
- Convert the formed set into array.
- Print elements of Set.
How remove duplicates from a list in typescript?
“remove duplicates from list typescript” Code Answer’s
- function uniq(a) {
- return Array. from(new Set(a));
- }