How do you pass a string in recursion?
Table of Contents
How do you pass a string in recursion?
Explanation: Recursive function (reverse) takes string pointer (str) as input and calls itself with next location to passed pointer (str+1). Recursion continues this way when the pointer reaches ‘\0’, all functions accumulated in stack print char at passed location (str) and return one by one.
What is recursion in Java with example?
In Java, a method that calls itself is known as a recursive method. And, this process is known as recursion. A physical world example would be to place two parallel mirrors facing each other. Any object in between them would be reflected recursively.
How do you find a substring in a string using recursion?
Take the input strings as character arrays Str and subStr. Function match(char *str1, char *substr1) takes two substrings and returns 1 if substr1 and str1 are the same. Both pointers point to characters present in strings, initially at starting positions. If substr is empty, then return 0.
How recursive function works in Java?

A recursive function calls itself, the memory for the called function is allocated on top of memory allocated to calling function and different copy of local variables is created for each function call.
What is recursive string?
Recursion in Java is a process in which a method calls itself continuously. In the programming language, if a program allows us to call a function inside the same function name, it is known as a recursive call of the function.
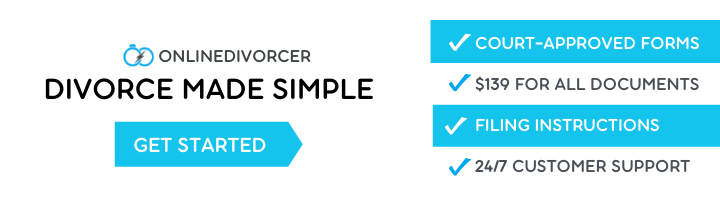
How do Substrings work in Java?
Substring in Java is a commonly used method of java. lang. String class that is used to create smaller strings from the bigger one. As strings are immutable in Java, the original string remains as it is, and the method returns a new string.
How can a given string be reversed using recursion in Java?
ReverseStringExample2.java
- class ReverseStringExample2.
- {
- //recursive function to reverse a string.
- void reverseString(String string)
- {
- if ((string==null)||(string.length() <= 1))
- System.out.println(string);
- else.
What is string copyValueOf () method in Java?
Java String copyValueOf() Method The copyValueOf() method returns a String that represents the characters of a char array. This method returns a new String array and copies the characters into it.
How are strings handled in Java?
In Java programming language, strings are treated as objects. The Java platform provides the String class to create and manipulate strings. String greeting = “Hello world!”; Whenever it encounters a string literal in your code, the compiler creates a String object with its value in this case, “Hello world!
Is Java recursion slow?
Recursion is slower and it consumes more memory since it can fill up the stack. But there is a work-around called tail-call optimization which requires a little more complex code (since you need another parameter to the function to pass around) but is more efficient since it doesn’t fill the stack.
Why recursive algorithms are inefficient?
Recursive algorithms are often inefficient for small data, due to the overhead of repeated function calls and returns. For this reason efficient implementations of recursive algorithms often start with the recursive algorithm, but then switch to a different algorithm when the input becomes small.
Is recursion hard to learn?
Recursion is not hard, whereas thinking recursively might be confusing in some cases. The recursive algorithm has considerable advantages over identical iterative algorithm such as having fewer code lines and reduced use of data structures.
What is recursion give example?
Recursion is the process of defining a problem (or the solution to a problem) in terms of (a simpler version of) itself. For example, we can define the operation “find your way home” as: If you are at home, stop moving. Take one step toward home.
How do I reverse a string in Java?
Objects of String are immutable.
What are the string methods in Java?
charAt ( position)
What is recursion method in Java?
– In the above program, main () is invoking nums () with argument 1. – This 1 comes to the method nums () into x. there 1 is printed. – Then nums () invokes itself with x+1 as argument. – It means 2 is passed this time to nums () as argument and x receives 2. – So 2 is printed, and then nums () is called with value 3.
How can I compare two strings in Java?
– Using equals () method (comparing the content) – Using == operator (comparing the object reference) – Using compareTo () method (comparing strings lexicographically)