How do you find a recursive prime number?
Table of Contents
How do you find a recursive prime number?
The goal is to find whether the input number Num is a prime or non-prime using recursion. To check if a number is prime or not, start traversing from i=2 to i<=Num/2. If any i is fully divisible by Num then the number is non-prime as prime numbers are only divisible by 1 and the number itself.
Is the set of prime numbers recursive?
For example, the set of primes is recursive, since we can determine whether a given number is prime by dividing it by all natural numbers less than or equal to its square root. With a bit of work, one can find a recursive function f to do the job.
How do you code a prime number in Python?
Method 1: Using isprime() to check if a number is prime or not in python

- 1.1 Code. def isprime(num): for n in range ( 2 , int (num * * 0.5 ) + 1 ): if num % n = = 0 :
- 1.2 Code. def isprime(num): if num = = 2 or num = = 3 :
- 1.3 Code. def isprime(num): if num = = 2 or num = = 3 :
- 1.4 Code. def isprime(num): if num> 1 :
What is prime number program?
Program to Check Prime Number Enter a positive integer: 29 29 is a prime number. In the program, a for loop is iterated from i = 2 to i < n/2 . If n is perfectly divisible by i , n is not a prime number. In this case, flag is set to 1, and the loop is terminated using the break statement.
How do you figure out if a number is prime?
If a number has only two factors 1 and itself, then the number is prime.
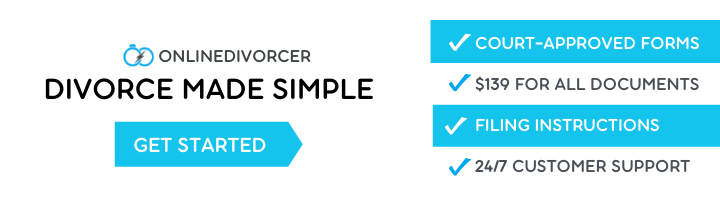
Is there a prime function in Python?
Python Function to Check for Prime Number The above function is_prime() takes in a positive integer n as the argument. If you find a factor in the specified range of (2, n-1), the function returns False —as the number is not prime. And it returns True if you traverse the entire loop without finding a factor.
How do you write a program for a prime number?
In this c program, we will take an input from the user and check whether the number is prime or not.
- #include
- int main(){
- int n,i,m=0,flag=0;
- printf(“Enter the number to check prime:”);
- scanf(“%d”,&n);
- m=n/2;
- for(i=2;i<=m;i++)
- {
How recursive function works in Python?
Recursive Functions in Python A recursive function is a function defined in terms of itself via self-referential expressions. This means that the function will continue to call itself and repeat its behavior until some condition is met to return a result.
What is recursive function in python with example?
In the above example, factorial() is a recursive function as it calls itself. When we call this function with a positive integer, it will recursively call itself by decreasing the number. Each function multiplies the number with the factorial of the number below it until it is equal to one.
How do you check a number is prime or not in Python?
for j in range(2, int(a/2) + 1): # If the given number is divisible or not. if (a % j) == 0: print(a, “is not a prime number”)
Is prime function Python efficient?
Function isPrime2 is faster in returning True for prime numbers. But if a number is big and it is not prime, it takes too long to return a value. First function works better with that.
How do you print the first 10 prime numbers in Python?
Program Code
- numr=int(input(“Enter range:”))
- print(“Prime numbers:”,end=’ ‘)
- for n in range(1,numr):
- for i in range(2,n):
- if(n%i==0):
- break.
- else:
- print(n,end=’ ‘)
What is recursion in Python give example?
Python also accepts function recursion, which means a defined function can call itself. Recursion is a common mathematical and programming concept. It means that a function calls itself. This has the benefit of meaning that you can loop through data to reach a result.