How do you declare a string literal?
Table of Contents
How do you declare a string literal?
The best way to declare a string literal in your code is to use array notation, like this: char string[] = “I am some sort of interesting string. \n”; This type of declaration is 100 percent okey-doke.
Is it possible to modify a string literal in C?
C Language Undefined behavior Modify string literal Attempting to modify the string literal has undefined behavior. However, modifying a mutable array of char directly, or through a pointer is naturally not undefined behavior, even if its initializer is a literal string.
What is a literal string give an example?
A string literal is a sequence of zero or more characters enclosed within single quotation marks. The following are examples of string literals: ‘Hello, world!’ ’10-NOV-91′ ‘He said, “Take it or leave it.”‘

What type is a string literal in C?
In C the type of a string literal is a char[]. In C++, an ordinary string literal has type ‘array of n const char’. For example, The type of the string literal “Hello” is “array of 6 const char”. It can, however, be converted to a const char* by array-to-pointer conversion.
How many ways can you write a literal string in a program?
A string literal can be specified in four different ways:
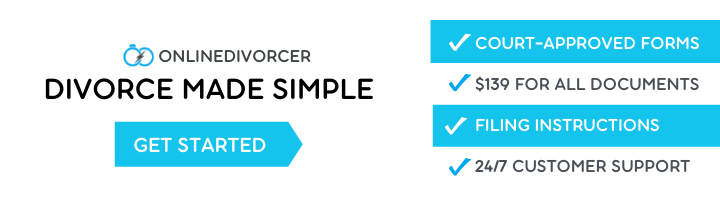
- single quoted.
- double quoted.
- heredoc syntax.
- nowdoc syntax.
Can we assign string to char pointer?
When you say char * str1 in C, you are allocating a pointer in the memory. When you write str1 = “Hello”; , you are creating a string literal in memory and making the pointer point to it. When you create another string literal “new string” and assign it to str1 , all you are doing is changing where the pointer points.
Is a string literal a pointer?
String literals are stored just like arrays. The most important point to understand is that a string literal is a pointer to the first character of the array. In other words “Hello World” is a pointer to the character ‘H’ .
What is a literal string in C?
A “string literal” is a sequence of characters from the source character set enclosed in double quotation marks (” “). String literals are used to represent a sequence of characters which, taken together, form a null-terminated string.
What means literal string?
Alternatively referred to as literal(s), a literal string is a series of characters enclosed in double or single quotes, depending on the programming language or command line. A program does not interpret characters in a literal string until it encounters the next double or single quote.
Where are string literals stored?
String literals are stored in C as an array of chars, terminted by a null byte. A null byte is a char having a value of exactly zero, noted as ‘\0’.
What are examples of string literals in c?
String literals are stored in C as an array of chars, terminted by a null byte….String literals may be subscripted:
- “abc” [ 2 ] results in ‘c’
- “abc” [ 0 ] results in ‘a’
- “abc” [ 3 ] results in the null byte.
Are string literals pointers?
String literal as a Pointer String literals are stored just like arrays. The most important point to understand is that a string literal is a pointer to the first character of the array.
How do I store string in char pointer?
C
- Strings using character pointers. Using character pointer strings can be stored in two ways:
- 1) Read only string in a shared segment.
- 2) Dynamically allocated in heap segment.
- Example 1 (Try to modify string)
- Example 2 (Try to return string from a function)
What is string literal in C?
What are the two types of string literals?
A string literal with the prefix L is a wide string literal. A string literal without the prefix L is an ordinary or narrow string literal. The type of narrow string literal is array of char . The type of a wide character string literal is array of wchar_t Both types have static storage duration.
What is a literal string value?
A string is a sequence of characters. The literal value for a string is written by surrounding the value with quotes or apostrophes. There are several variations to provide some additional features. Basic String.
Which is better string literal or new keyword?
In general, we should use the String literal notation when possible. It is easier to read and it gives the compiler a chance to optimize our code.