How do you convert int to float value?
Table of Contents
How do you convert int to float value?
int to float conversion in Java
- public class IntToFloatExample {
- public static void main(String args[])
- {
- int i1 = 10;
- float f1 = i1;
- System.out.println(“int value: ” + i1);
- System.out.println(“Converted float value: ” + f1);
- }
Can integer convert to float?
To convert an integer data type to float you can wrap the integer with float64() or float32. Explanation: Firstly we declare a variable x of type int64 with a value of 5. Then we wrap x with float64(), which converts the integer 5 to float value of 5.00. The %.
Can you convert int to float in C?
You can’t reassign i as a float after that. An int is always an int and will remain an int as long as it was declared as an int and will never be able to change into anything but an int. What you did was type casting, but because your left value was an int the resulting value was an int.

What happens when float is converted to int?
To convert a float value to int we make use of the built-in int() function, this function trims the values after the decimal point and returns only the integer/whole number part. Example 1: Number of type float is converted to a result of type int.
How do you convert a variable to a float?
We can convert a string to float in Python using float() function. It’s a built-in function to convert an object to floating point number. Internally float() function calls specified object __float__() function.
Can you store an int in a float?
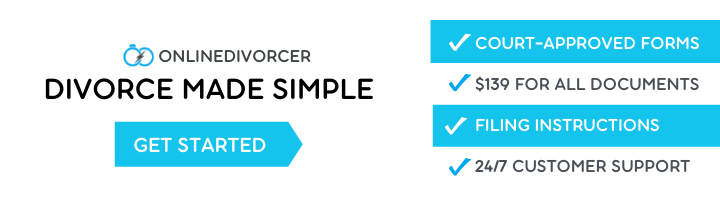
So you cannot store a float value in an int object through simple assignment. You can store the bit pattern for a floating-point value in an int object if the int is at least as wide as the float , either by using memcpy or some kind of casting gymnastics (as other answers have shown).
How does the int function convert a float to an int?
How does the int function convert a float to an int? By rounding to the nearest whole number.
Why it is often a best practice to convert floating point values to integers when performing arithmetic operations?
First, they can represent values between integers. Second, because of the scaling factor, they can represent a much greater range of values. On the other hand, floating point operations usually are slightly slower than integer operations, and you can lose precision.
Can we convert int to float in Java?
So, when you assign an int value to float variable, the conversion of int to float automatically happens in Java. This is also called widening casting or widening primitive conversion. So, to convert an in to float using widening casting, you can just assign a value of int to float.
How do you turn a string into a float?
Let’s see the simple example of converting String to float in java.
- public class StringToFloatExample{
- public static void main(String args[]){
- String s=”23.6″;
- float f=Float.parseFloat(“23.6”);
- System.out.println(f);
- }}
How do you store float value?
Scalars of type float are stored using four bytes (32-bits). The format used follows the IEEE-754 standard. The mantissa represents the actual binary digits of the floating-point number.
Can we store int value in float Java?
float has 24 bits of precision (and a sign bit) which means you can store int values between -16777216 to 16777216 without loss of precision.
How do you do implicit type conversion?
We perform the implicit typecasting by passing the value of a variable into a different type of variable.
- We pass the value of variable p to variable q that is of type short.
- We pass the value of variable q to variable r that is of type int.
- We pass the value of variable r to variable s that is of type long.
Why it is often a best practice to convert floating-point values to integers when performing arithmetic operations?
What is the advantage of normalized floating point number?
A normalized number provides more accuracy than corresponding de-normalized number. The implied most significant bit can be used to represent even more accurate significant (23 + 1 = 24 bits) which is called subnormal representation. The floating point numbers are to be represented in normalized form.