How do you calculate Fibonacci in Java?
Table of Contents
How do you calculate Fibonacci in Java?
Fibonacci Series using recursion in java
- class FibonacciExample2{
- static int n1=0,n2=1,n3=0;
- static void printFibonacci(int count){
- if(count>0){
- n3 = n1 + n2;
- n1 = n2;
- n2 = n3;
- System.out.print(” “+n3);
What are Fibonacci Series in Java?
The Fibonacci series is a series where the next term is the sum of the previous two terms. The first two terms of the Fibonacci sequence are 0 followed by 1. Fibonacci Series: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34.
What is Fibonacci example?

Fibonacci Sequence = 0, 1, 1, 2, 3, 5, 8, 13, 21, …. “3” is obtained by adding the third and fourth term (1+2) and so on. For example, the next term after 21 can be found by adding 13 and 21. Therefore, the next term in the sequence is 34.
How do you print Fibonacci numbers?
Fibonacci Series in C without recursion
- #include
- int main()
- {
- int n1=0,n2=1,n3,i,number;
- printf(“Enter the number of elements:”);
- scanf(“%d”,&number);
- printf(“\n%d %d”,n1,n2);//printing 0 and 1.
- for(i=2;i
What is fibonacci series using recursion in Java?
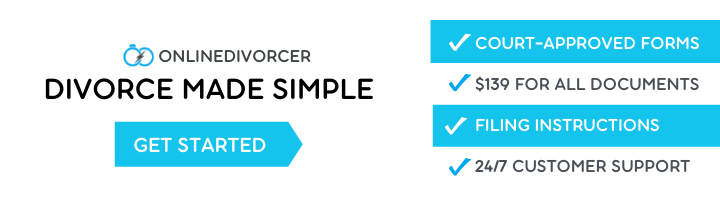
Fibonacci Series Using Recursion in Java The Java Fibonacci recursion function takes an input number. Checks for 0, 1, 2 and returns 0, 1, 1 accordingly because Fibonacci sequence in Java starts with 0, 1, 1. When input n is >=3, The function will call itself recursively. The call is done two times.
What is formula of Fibonacci?
The Fibonacci formula is given as, Fn = Fn-1 + Fn-2, where n > 1.
How do you find the N in a Fibonacci sequence?
the n-th Fibonacci number is the sum of the (n-1)th and the (n-2)th. So to calculate the 100th Fibonacci number, for instance, we need to compute all the 99 values before it first – quite a task, even with a calculator!
What is the Fibonacci of 2?
The notation that we will use to represent the Fibonacci sequence is as follows: f1=1,f2=1,f3=2,f4=3,f5=5,f6=8,f7=13,f8=21,f9=34,f10=55,f11=89,f12=144,…
How do you write Fibonacci in recursion?
Code : Compute fibonacci numbers using recursion method
- #include
- int Fibonacci(int);
- int main()
- int n, i = 0, c;
- scanf(“%d”,&n);
- printf(“Fibonacci series\n”);
- for ( c = 1 ; c <= n ; c++ )
- {
How Fibonacci recursive method works?
Recursion will happen till the bottom of each branch in the tree structure is reached with the resulting value of 1 or 0. During recursion these 1’s and 0’s are added till the value of the Fibonacci number is calculated and returned to the code which called the fibonacci method in the first place.
What is the Fibonacci of 4?
3
Fibonacci Numbers List
F0 = 0 | F10 = 55 |
---|---|
F3 = 2 | F13 = 233 |
F4 = 3 | F14 = 377 |
F5 = 5 | F15 = 610 |
F6 = 8 | F16 = 987 |
How do you find the nth number in a Fibonacci sequence in Java?
- { // Function to find the nth Fibonacci number. public static int fib(int n)
- { if (n <= 1) { return n;
- } return fib(n – 1) + fib(n – 2);
- } public static void main(String[] args)
- { int n = 8;
- System. out. println(“F(n) = ” + fib(n)); } }
How do you find the nth term of a Fibonacci sequence in Java?
So to find the nth, you have to iterate the step n times: for (loop = 0; loop < n; loop ++) { fibonacci = num + num2; num = num2; num2 = fibonacci; } System. out. print(num);