How do I resolve a NullPointerException?
Table of Contents
How do I resolve a NullPointerException?
In Java, the java. lang. NullPointerException is thrown when a reference variable is accessed (or de-referenced) and is not pointing to any object. This error can be resolved by using a try-catch block or an if-else condition to check if a reference variable is null before dereferencing it.
Which of the following options can throw a NullPointerException?
NullPointerException is thrown when program attempts to use an object reference that has the null value. These can be: Invoking a method from a null object. Accessing or modifying a null object’s field.
How can we avoid NullPointerException in Java with example?
Answer: Some of the best practices to avoid NullPointerException are:

- Use equals() and equalsIgnoreCase() method with String literal instead of using it on the unknown object that can be null.
- Use valueOf() instead of toString() ; and both return the same result.
- Use Java annotation @NotNull and @Nullable.
Why do we get NullPointerException?
NullPointerException is a runtime exception and it is thrown when the application try to use an object reference which has a null value. For example, using a method on a null reference.
Is NullPointerException a runtime exception?
A possible reason because a NullPointerException is a runtime exception is because every method can throw it, so every method would need to have a “throws NullPointerException”, and would be ugly.
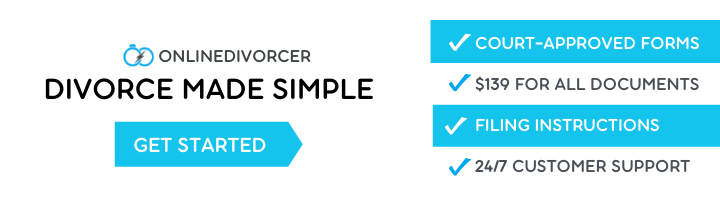
How do you write not null in JavaScript?
==) operator to check if a variable is not null – myVar !== null . The strict inequality operator will return true if the variable is not equal to null and false otherwise.
How do you stop a null check in Java?
10 Tips to Handle Null Effectively
- Don’t Overcomplicate Things.
- Use Objects Methods as Stream Predicates.
- Never Pass Null as an Argument.
- Validate Public API Arguments.
- Return Empty Collections Instead of Null.
- Optional Ain’t for Fields.
- Use Exceptions Over Nulls.
- Test Your Code.
What do you understand by null pointer?
A null pointer has a reserved value that is called a null pointer constant for indicating that the pointer does not point to any valid object or function. You can use null pointers in the following cases: Initialize pointers. Represent conditions such as the end of a list of unknown length.
What is NullPointerException when it occurs?
NullPointerException s are exceptions that occur when you try to use a reference that points to no location in memory (null) as though it were referencing an object. Calling a method on a null reference or trying to access a field of a null reference will trigger a NullPointerException .
How do I use isNull in JavaScript?
isNull() function: It is used to find whether the value of the object is null. If object has null value then the output will be true otherwise false. We can even perform addition, subtraction etc operations in this function.
How do you null an object in JavaScript?
null is a primitive value that represents the intentional absence of any object value. If you see null (either assigned to a variable or returned by a function), then at that place should have been an object, but for some reason, an object wasn’t created. greetObject(‘Eric’); // => { message: ‘Hello, Eric!’ }
Why is NaN a number?
NaN stands for Not a Number. It is a value of numeric data types (usually floating point types, but not always) that represents the result of an invalid operation such as dividing by zero. Although its names says that it’s not a number, the data type used to hold it is a numeric type.
How can we avoid null pointer exception in Java with example?
How do you write null in Java?
Let’s see an example to provide null to the String variable.
- public class NullExample5 {
- static String str=null;
- public static void main(String[] args) {
- if(str==null)
- {
- System.out.println(“value is null”);
- }
- else.
How do you create a null pointer?
Declare a pointer p of the integer datatype. Initialize *p= NULL. Print “The value of pointer is”. Print the value of the pointer p.