How do I read an int file?
Table of Contents
How do I read an int file?
We use the getw() and putw() I/O functions to read an integer from a file and write an integer to a file respectively. Syntax of getw: int num = getw(fptr); Where, fptr is a file pointer.
How do you print and read a variable?
Steps:
- The user enters an integer value when asked.
- This value is taken from the user with the help of scanf() method.
- For an integer value, the X is replaced with type int.
- This entered value is now stored in the variableOfIntType.
- Now to print this value, printf() method is used.
How do you read an integer value in a program give example?
Code

- Reading an integer and a floating point value. #include int main() { int a; float b; int x = scanf(“%d%f”, &a, &b); printf(“Decimal Number is : %d\n”,a);
- Reading a string. #include int main() { char name[20]; scanf(“%s”, &name); printf(“Your name is: %s”, name); return 0;
What does Fscanf return in C?
The fscanf() function returns the number of fields that it successfully converted and assigned. The return value does not include fields that the fscanf() function read but did not assign. The return value is EOF if an input failure occurs before any conversion, or the number of input items assigned if successful.
How do you read integer value from keyboard?
To read integers from console, use Scanner class. Scanner myInput = new Scanner( System.in ); Allow a use to add an integer using the nextInt() method. System.
How do I read fscanf?
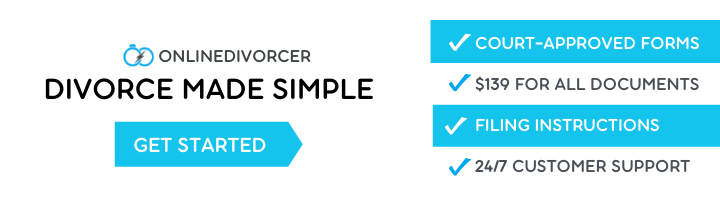
fscanf Function in C This function is used to read the formatted input from the given stream in the C language. Syntax: int fscanf(FILE *ptr, const char *format.) fscanf reads from a file pointed by the FILE pointer (ptr), instead of reading from the input stream.
How do I get fscanf to read newline?
- If the nameN fields cannot contain whitespace, just add a space to the front of the format string – ” %[^=]=%[^;];” – to skip leading whitespace.
- The accepted answer is right, anyway, about repeated newlines you could use %*[\n] to read an arbitrary number of ‘\n’ without storing them.
How do you read a vector in CPP?
In this tutorial, we will learn about vectors in C++ with the help of examples. In C++, vectors are used to store elements of similar data types….C++ Vector Functions.
Function | Description |
---|---|
front() | returns the first element of the vector |
back() | returns the last element of the vector |
How do I read a CPP file?
In order for your program to read from the file, you must:
- include the fstream header file with using std::ifstream;
- declare a variable of type ifstream.
- open the file.
- check for an open file error.
- read from the file.
- after each read, check for end-of-file using the eof() member function.
Which Scanner class method is used to read integer value from the user?
nextInt()
Scanner Class Methods
Method | Description |
---|---|
nextInt() | Reads an int value from the user |
nextLine() | Reads a String value from the user |
nextLong() | Reads a long value from the user |
nextShort() | Reads a short value from the user |