Can you filter an array in Java?
Table of Contents
Can you filter an array in Java?
You can use List. removeAll() method to filter an array.
How do you filter an array of objects?
One can use filter() function in JavaScript to filter the object array based on attributes. The filter() function will return a new array containing all the array elements that pass the given condition. If no elements pass the condition it returns an empty array.
How do you filter a list in Java?

Java 8 (2014) solves this problem using streams and lambdas in one line of code:
- List beerDrinkers = persons. stream() . filter(p -> p. getAge() > 16). collect(Collectors.
- persons. removeIf(p -> p. getAge() <= 16);
- List beerDrinkers = select(persons, having(on(Person. class). getAge(), greaterThan(16)));
How do you set a filter in Java?
You can filter Java Collections like List, Set or Map in Java 8 by using the filter() method of the Stream class. You first need to obtain a stream from Collection by calling stream() method and then you can use the filter() method, which takes a Predicate as the only argument.
How do you filter only one property from an array of objects?
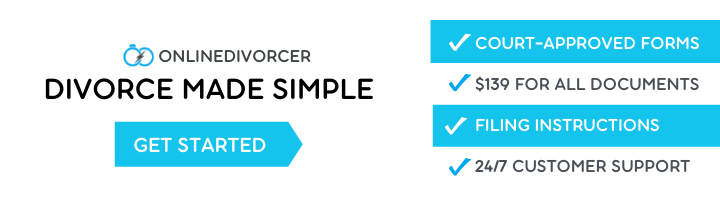
To filter an array of objects based on a property:
- Call the Array. filter method passing it a function.
- The function will be called with each element of the array and should do a conditional check on the property.
- The Array. filter methods returns an array with all elements that satisfy the condition.
How do you filter multiple objects in an array?
To filter an array with multiple conditions:
- Call the Array. filter method, passing it a function.
- The function should use the && (And) operator to check for the conditions.
- Array. filter returns all elements that satisfy the conditions.
What is filters in Java?
The Java Servlet specification version 2.3 introduces a new component type, called a filter. A filter dynamically intercepts requests and responses to transform or use the information contained in the requests or responses.
What is filter method in Java?
The filter() function of the Java stream allows you to narrow down the stream’s items based on a criterion. If you only want items that are even on your list, you can use the filter method to do this. This method accepts a predicate as an input and returns a list of elements that are the results of that predicate.
What is the difference between node and filter streams?
Node is more like nodeJS while filter is just String function filtering out texts.
How does filter works in Java?
filter() is a intermediate Stream operation. It returns a Stream consisting of the elements of the given stream that match the given predicate. The filter() argument should be stateless predicate which is applied to each element in the stream to determine if it should be included or not.
How do you filter a string in Java?
Filter a String in Java
- Using String. replaceAll() method.
- Using Java 8. In Java 8 and above, use chars() or codePoints() method of String class to get an IntStream of char values from the given sequence.
- Using CharMatcher by Guava. Several third-party libraries provide utility methods for working with strings.
How do you filter an array of numbers?
Use the filter() method to filter an array to only numbers, e.g. arr. filter(value => typeof value === ‘number’) . The filter method returns an array with all the elements that satisfy the condition, in our case all array elements with a type of number .
How do you filter two conditions?
If you want to put multiple conditions in filter , you can use && and || operator.
What and why filters are used in Java?
A filter is an object that is invoked at the preprocessing and postprocessing of a request. It is mainly used to perform filtering tasks such as conversion, logging, compression, encryption and decryption, input validation etc. The servlet filter is pluggable, i.e. its entry is defined in the web.
What is the difference between filter and map in Java?
Filter takes a predicate as an argument so basically you are validating your input/collection against a condition, whereas a map allows you to define or use a existing function on the stream eg you can apply String.
What is filter method Java 8?
Java 8 Stream interface introduces filter() method which can be used to filter out some elements from object collection based on a particular condition. This condition should be specified as a predicate which the filter() method accepts as an argument.