Can I use forEach on object?
Table of Contents
Can I use forEach on object?
JavaScript’s Array#forEach() function lets you iterate over an array, but not over an object. But you can iterate over a JavaScript object using forEach() if you transform the object into an array first, using Object. keys() , Object.
How do you iterate an object object?
How to iterate over object properties in JavaScript
- const items = { ‘first’: new Date(), ‘second’: 2, ‘third’: ‘test’ }
- items. map(item => {})
- items. forEach(item => {})
- for (const item of items) {}
- for (const item in items) { console. log(item) }
- Object. entries(items). map(item => { console. log(item) }) Object.
Can you loop through an object JavaScript?
Object. key(). It returns the values of all properties in the object as an array. You can then loop through the values array by using any of the array looping methods.

How do I iterate an object in node JS?
Since Javascript 1.7 there is an Iterator object, which allows this: var a={a:1,b:2,c:3}; var it=Iterator(a); function iterate(){ try { console. log(it. next()); setTimeout(iterate,1000); }catch (err if err instanceof StopIteration) { console.
How do you iterate in JavaScript?
- There are multiple ways one can iterate over an array in Javascript. The most useful ones are mentioned below.
- Using while loop. This is again similar to other languages.
- using forEach method.
- Using every method.
- Using map.
- Using Filter.
- Using Reduce.
- Using Some.
How do you filter an object in JavaScript?
keys() to filter an Object. After you’ve generated the keys, you may use filter() to loop over the existing values and return just those that meet the specified criteria. Finally, you can use reduce() to collect the filtered keys and their values into a new object, for instance.
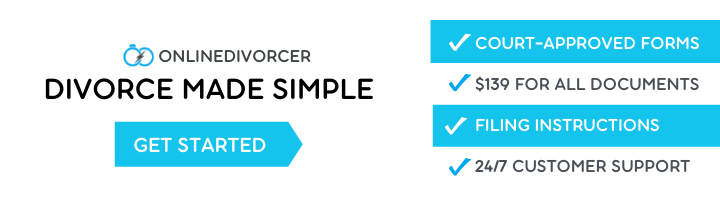
How do I iterate an array of objects in Node JS?
forEach() is an array function from Node. js that is used to iterate over items in a given array. Parameter: This function takes a function (which is to be executed) as a parameter. Return type: The function returns array element after iteration.
How do you iterate keys in JavaScript?
The Object. keys() method was introduced in ES6. It takes the object that you want to iterate over as an argument and returns an array containing all properties names (or keys). You can then use any of the array looping methods, such as forEach(), to iterate through the array and retrieve the value of each property.
Is JS forEach sequential?
forEach calls a provided callback function once for each element in an array in ascending order. Callback runs in sequential but it does not wait until one is finished. That is because it runs not like iteration which runs next step when one step is finished.
Is forEach faster than for?
Deductions. This foreach loop is faster because the local variable that stores the value of the element in the array is faster to access than an element in the array. The forloop is faster than the foreach loop if the array must only be accessed once per iteration.
How do I run a forEach loop only in JavaScript?
There is, just put a break at the end of the block. But instead of doing this just get the one item you want out of the collection instead of “looping once”. foreach traverses each element once it does not loop.
How do you filter keys for objects?
JavaScript objects don’t have a filter() method, you must first turn the object into an array to use array’s filter() method. You can use the Object. keys() function to convert the object’s keys into an array, and accumulate the filtered keys into a new object using the reduce() function as shown below.
How do you filter an array of objects in JavaScript based on property value?
To filter an array of objects based on a property:
- Call the Array. filter method passing it a function.
- The function will be called with each element of the array and should do a conditional check on the property.
- The Array. filter methods returns an array with all elements that satisfy the condition.
How do you use each in JavaScript?
each(), which is used to iterate, exclusively, over a jQuery object. The $. each() function can be used to iterate over any collection, whether it is an object or an array. In the case of an array, the callback is passed an array index and a corresponding array value each time.