Are objects created on the stack C++?
Table of Contents
Are objects created on the stack C++?
Unlike Java, instances of classes (“objects”) can be allocated on the stack in C++.
How do you declare an object with a constructor in C++?
There are two ways to initialize a class object:
- Using a parenthesized expression list. The compiler calls the constructor of the class using this list as the constructor’s argument list.
- Using a single initialization value and the = operator.
Can we call constructor using object in C++?
Constructor is a member function of class, whose name is same as the class. A constructor is a special type of member function of a class which initializes objects of a class. In C++, Constructor is automatically called when object(instance of class) is created. Constructor is invoked at the time of object creation.

Can constructors have objects as parameters?
A Java class constructor initializes instances (objects) of that class. Typically, the constructor initializes the fields of the object that need initialization. Java constructors can also take parameters, so fields can be initialized in the object at creation time.
Are objects created on the stack?
Static (and thread-local) objects are generally allocated in their own memory regions, neither on the stack nor on the heap. And member variables are allocated wherever the object they belong to is allocated.
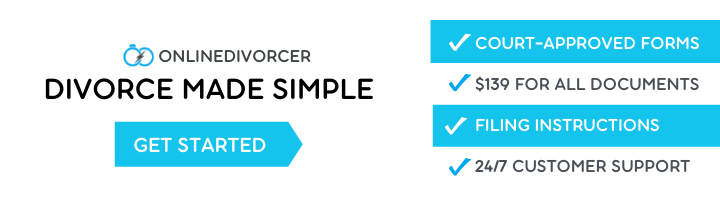
Are objects stored on stack?
Yes, an object can be stored on the stack. If you create an object inside a function without using the “new” operator then this will create and store the object on the stack, and not on the heap.
Can an object be created inside constructor?
We can write ‘return’ inside a constructor. Now the most important topic that comes into play is the strong incorporation of OOPS with constructors known as constructor overloading. JustLike methods, we can overload constructors for creating objects in different ways.
Can we call constructor using object?
No, you cannot call a constructor from a method. The only place from which you can invoke constructors using “this()” or, “super()” is the first line of another constructor. If you try to invoke constructors explicitly elsewhere, a compile time error will be generated.
Does constructor create object?
Simply speaking, a constructor does not create an object. It just initializes the state of the object. It’s the new operator which creates the object.
Can an object be on the stack?
Are objects created on stack or heap?
The Heap Space contains all objects are created, but Stack contains any reference to those objects. Objects stored in the Heap can be accessed throughout the application. Primitive local variables are only accessed the Stack Memory blocks that contain their methods.
Where are C++ objects allocated?
All dynamic allocations are on the heap.
Can we create an object without using its constructor?
Actually, yes, it is possible to bypass the constructor when you instantiate an object, if you use objenesis to instantiate the object for you. It does bytecode manipulations to achieve this. Deserializing an object will also bypass the constructor.
Can we initialize object without constructor?
You can use object initializers to initialize type objects in a declarative manner without explicitly invoking a constructor for the type.
How do you instantiate an object in C++?
Instantiating a Class The new operator requires a single, postfix argument: a call to a constructor. The name of the constructor provides the name of the class to instantiate. The constructor initializes the new object. The new operator returns a reference to the object it created.
Can we call constructor without creating object?
NO. You can’t invoke a constructor without creating an object.
Can we call the constructor more than once for object?
Constructors are called only once at the time of the creation of the object.
What is the object in constructor?
The Object constructor creates an object wrapper for the given value. If the value is null or undefined , it will create and return an empty object. Otherwise, it will return an object of a Type that corresponds to the given value. If the value is an object already, it will return the value.
Do objects reside in stack memory?
New objects are always created in heap space, and the references to these objects are stored in stack memory. These objects have global access and we can access them from anywhere in the application.
What is the use of constructor in stack?
This constructor is an O (1) operation. Initializes a new instance of the Stack class that contains elements copied from the specified collection and has the same initial capacity as the number of elements copied. The ICollection to copy elements from.
How do you create a new object on the stack?
The first line creates a new object on the stack by calling a constructor of the format Thing (const char*). The second one is a bit more complex. It essentially does the following
How to create a copy of the stack in Java?
The Stack constructor is used again to create a copy of the stack with the order of elements reversed; thus, the three null elements are at the end. The Contains method is used to show that the string “four” is in the first copy of the stack, after which the Clear method clears the copy and the Count property shows that the stack is empty.
What is a stack variable in C programming?
Stack in C can store pointers, or fixed arrays or structs – all of fixed size, and these things are being allocated in memory in linear order. When a C program frees a stack variable – it just moves stack pointer back and nothing more.