What to do if Cannot find symbol in Java?
Table of Contents
What to do if Cannot find symbol in Java?
In the above program, “Cannot find symbol” error will occur because “sum” is not declared. In order to solve the error, we need to define “int sum = n1+n2” before using the variable sum.
Can you add to an ArrayList in Java?
We can add or remove elements anytime. So, it is much more flexible than the traditional array. Element can be added in Java ArrayList using add() method of java.
How do you add to ArrayList?
To add an object to the ArrayList, we call the add() method on the ArrayList, passing a pointer to the object we want to store. This code adds pointers to three String objects to the ArrayList… list. add( “Easy” ); // Add three strings to the ArrayList list.

How do you represent an ArrayList in Java?
Java ArrayList Example
- import java.util.*;
- public class ArrayListExample1{
- public static void main(String args[]){
- ArrayList list=new ArrayList();//Creating arraylist.
- list.add(“Mango”);//Adding object in arraylist.
- list.add(“Apple”);
- list.add(“Banana”);
- list.add(“Grapes”);
What does Cannot find symbol mean?
Any error that starts “cannot find symbol” means that the compiler doesn’t know what that symbol (which can be a variable or a class name) refers to. In the second line of the error, where it says “symbol: class Scanner”, that indicates that it doesn’t know what the Scanner class is.
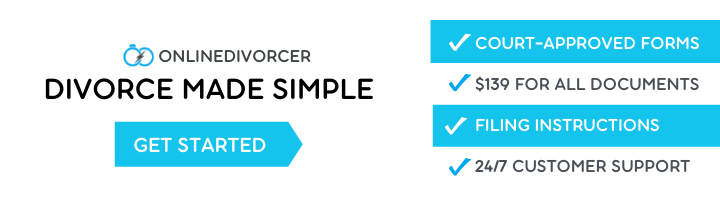
What is ADD () in Java?
The java. util. ArrayList. add(int index, E elemen) method inserts the specified element E at the specified position in this list.It shifts the element currently at that position (if any) and any subsequent elements to the right (will add one to their indices).
Can we add object in ArrayList?
To add an element or object to Java ArrayList, use ArrayList. add() method. ArrayList. add(element) method appends the element or object to the end of ArrayList.
How do you add an element to a list in Java?
There are two methods to add elements to the list.
- add(E e): appends the element at the end of the list. Since List supports Generics, the type of elements that can be added is determined when the list is created.
- add(int index, E element): inserts the element at the given index.
How do you add an array to an ArrayList in Java?
An array can be converted to an ArrayList using the following methods: Using ArrayList. add() method to manually add the array elements in the ArrayList: This method involves creating a new ArrayList and adding all of the elements of the given array to the newly created ArrayList using add() method.
What does illegal start of expression mean in Java?
Summary. To sum up, the “Illegal start of expression” error occurs when the Java compiler finds something inappropriate with the source code at the time of execution. To debug this error, try looking at the lines preceding the error message for missing brackets, curly braces, or semicolons and check the syntax.
How do I add a list to a list in Java?
You insert elements (objects) into a Java List using its add() method. Here is an example of adding elements to a Java List using the add() method: List listA = new ArrayList<>(); listA. add(“element 1”); listA.
How do you add an object to a list?
How do I add an item to a list in Java?
How do you add an array value to an ArrayList in Java?
Using Arrays. asList() method – Pass the required array to this method and get a List object and pass it as a parameter to the constructor of the ArrayList class. Collections. addAll() method – Create a new list before using this method and then add array elements using this method to existing list.