What is use of unassigned local variable in C#?
Table of Contents
What is use of unassigned local variable in C#?
Here is a simple tip that you may not have run across yet. TLDR: Initialize a variable to null to indicate to the compiler that you plan to assign it later.
Is Catch mandatory for try in C#?
It is not mandatory. The catch statement is the exception handler in the Try Catch statements. A try block can have multiple catch blocks. But it must be declared the Exception class is a final one.
Can we use catch ()’ without passing arguments in it?
Some exception can not be catch(Exception) catched. Below excecption in mono on linux, should catch without parameter. Otherwise runtime will ignore catch(Exception) statment.

What will happen if exception occurs in catch block in C#?
The Anatomy of C# Exceptions If any code throws an exception within that try block, the exception will be handled by the corresponding catch. catch – When an exception occurs, the Catch block of code is executed. This is where you are able to handle the exception, log it, or ignore it.
What is the value of an unassigned variable C#?
The value for an unitialized variable of type T is always default(T) . For all reference types this is null, and for the value types see the link that @Blorgbeard posted (or write some code to check it).
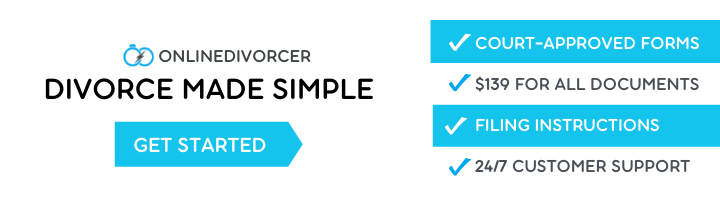
What is an unassigned variable?
In computing, an uninitialized variable is a variable that is declared but is not set to a definite known value before it is used.
How do you handle errors without try catch?
The best alternative I can give you is Elmah. http://code.google.com/p/elmah/ It will handle all your uncaught errors and log them. From there I would suggest fixing said errors or catching the specific errors you expect and not just catch any error that might occur.
Can we write finally without try catch?
Yes, it is not mandatory to use catch block with finally. You can have to try and finally.
Does try-catch stop execution?
The “try… First, the code in try {…} is executed. If there were no errors, then catch (err) is ignored: the execution reaches the end of try and goes on, skipping catch . If an error occurs, then the try execution is stopped, and control flows to the beginning of catch (err) .
Can we throw exception in catch block?
The caller has to handle the exception using a try-catch block or propagate the exception. We can throw either checked or unchecked exceptions. The throws keyword allows the compiler to help you write code that handles this type of error, but it does not prevent the abnormal termination of the program.
How do you handle exception thrown in catch block?
What is an unassigned variable in C?
An unassigned variable in C is not “an unknown”, though it is (as an adjective) unknown. It’s uninitialized and thus equal to some value though it must be initialized first in order to be useful since only then do we know how to predict its behavior.
What is the value of an unassigned int in C#?
0
The default value for int is 0.
What happens when the local variable is not initialized & used inside a program?
If the programmer, by mistake, did not initialize a local variable and it takes a default value, then the output could be some unexpected value. So in case of local variables, the compiler will ask the programmer to initialize it with some value before they access the variable to avoid the usage of undefined values.
How do you handle exceptions without using try and catch C#?
How to handle exception without try catch block in c# with example. You can get the exception on Application_Error method in Global. asax method like below. But keep in mind that it’s not an alternative way you can use instead of try catch methods to handle exceptions.
Why you should avoid try catch?
One reason to avoid #1 is that it poisons your ability to use exception breakpoints. Normal-functioning code will never purposefully trigger a null pointer exception. Thus, it makes sense to set your debugger to stop every time one happens. In the case of #1, you’ll probably get such exceptions routinely.
Is Catch mandatory in try-catch?
Yes you can write try without catch. In that case you require finally block. Try requires either catch or finally or both that is at least one catch or finally is compulsory.
Can we write try-catch inside finally block?
A try-finally block is possible without catch block. Which means a try block can be used with finally without having a catch block.
How do you continue code after try-catch?
If an exception is caught and not rethrown, the catch() clause is executed, then the finally() clause (if there is one) and execution then continues with the statement following the try/catch/finally block.