What is the use of KeyListener?
Table of Contents
What is the use of KeyListener?
Implementing the KeyListener Interface The KeyListener interface listens to key events and performs some action. The declaration of this interface is shown below. We need to override the following three methods of this interface in our class. The keyPressed(KeyEvent e) method will be invoked when a key is pressed.
What is source and listener?
A source generates an event and sends it to one or more listeners. Listener simply waits until it receives an event. Listener processes events and then returns.
How do I listen to a key in Java?
In short, in order to implement a simple key listener in Java, one should perform these steps:

- Create a new class that extends KeyAdapter class.
- Override the keyPressed method to customize the handling of that specific event. Now every time the user presses a key this method will be launched.
- Use KeyEvent.
How do I use the scanner keyboard in Java?
Input from the keyboard
- import java.util.Scanner; – imports the class Scanner from the library java.util.
- Scanner scanner = new Scanner(System.in); – creates a new Scanner object, that is connected to standard input (the keyboard)
- String inputString = scanner. nextLine();
What is key event in Java?
An event which indicates that a keystroke occurred in a component. This low-level event is generated by a component object (such as a text field) when a key is pressed, released, or typed.
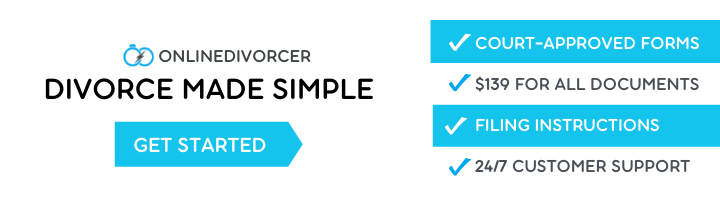
What are Adaptor classes in Java?
What Is An Adapter-Class? In JAVA, an adapter class allows the default implementation of listener interfaces. The notion of listener interfaces stems from the Delegation Event Model. It is one of the many techniques used to handle events in Graphical User Interface (GUI) programming languages, such as JAVA.
What is collection in Java Mcq?
What is Collection in Java? Explanation: A collection is a group of objects, it is similar to String Template Library (STL) of C++ programming language. Take Java Programming Practice Tests – Chapterwise!
How do you scan a string in Java?
Example 1
- import java.util.*;
- public class ScannerExample {
- public static void main(String args[]){
- Scanner in = new Scanner(System.in);
- System.out.print(“Enter your name: “);
- String name = in.nextLine();
- System.out.println(“Name is: ” + name);
- in.close();
How do I scan a double in Java?
Example 3
- import java.util.*;
- public class ScannerNextDoubleExample3 {
- public static void main(String args[]){
- Scanner scan = new Scanner(System.in);
- System.out.print(“Enter value: “);
- if(scan.hasNextDouble())
- {
- double y = scan.nextDouble();
How do you write a listener in Java?
How to write ActionListener
- Implement the ActionListener interface in the class: public class ActionListenerExample Implements ActionListener. public class ActionListenerExample Implements ActionListener.
- Register the component with the Listener: component.
- Override the actionPerformed() method:
What is adopter class?
Adapter class is a class that is defined using the adapter pattern. An adapter can be used to add new capabilities to an existing class without modifying the original class. For example, the java.