What is the algorithm of merge sort?
Table of Contents
What is the algorithm of merge sort?
Merge sort is a sorting algorithm based on the Divide and conquer strategy. It works by recursively dividing the array into two equal halves, then sort them and combine them. It takes a time of (n logn) in the worst case.
How do you program a merge sort?
Merge( ) Function Explained Step-By-Step
- Step 1: Create duplicate copies of sub-arrays to be sorted.
- Step 2: Maintain current index of sub-arrays and main array.
- Step 3: Until we reach the end of either L or M, pick larger among elements L and M and place them in the correct position at A[p..r]
How is merge sort algorithm calculated?
Merge sort algorithm steps

- Divide part: We calculate the mid-value, mid = l + (r – l)/2.
- Conquer part 1: We call the same function with mid as the right end and recursively sort the left part of size n/2, i.e., mergeSort(A, l, mid).
How do you write a merge sort algorithm in Java?
Program: Write a program to implement merge sort in Java.
- class Merge {
- /* Function to merge the subarrays of a[] */
- void merge(int a[], int beg, int mid, int end)
- {
- int i, j, k;
- int n1 = mid – beg + 1;
- int n2 = end – mid;
- /* temporary Arrays */
How is a merge sort algorithm implemented in C?
Merge Sort Algorithm
- Find the middle index of the array to divide it in two halves: m = (l+r)/2.
- Call MergeSort for first half: mergeSort(array, l, m)
- Call mergeSort for second half: mergeSort(array, m+1, r)
- Recursively, merge the two halves in a sorted manner, so that only one sorted array is left:
How do you code a merge sort in Python?
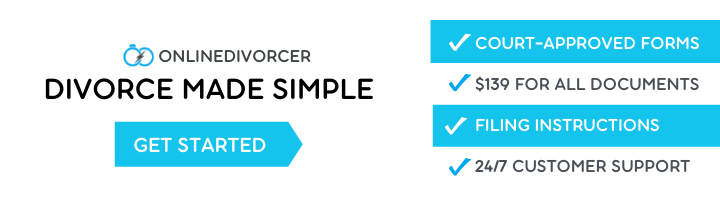
Implementation
- def mergeSort(myList):
- if len(myList) > 1:
- mid = len(myList) // 2.
- left = myList[:mid]
- right = myList[mid:]
-
- # Recursive call on each half.
- mergeSort(left)
Is merge sort in place algorithm?
The standard merge sort algorithm is an example of out-of-place algorithm as it requires O(n) extra space for merging. The merging can be done in-place, but it increases the time complexity of the sorting routine.
How do you write pseudocode algorithm?
Rules of writing pseudocode Have only one statement per line. Indent to show hierarchy, improve readability, and show nested constructs. Always end multiline sections using any of the END keywords (ENDIF, ENDWHILE, etc.). Keep your statements programming language independent.
How is merge sort complexity calculated?
Time complexity of Merge Sort is O(n*Log n) in all the 3 cases (worst, average and best) as merge sort always divides the array in two halves and takes linear time to merge two halves. It requires equal amount of additional space as the unsorted array.
How is a merge sort algorithm implemented in Python?
Merge sort works by splitting the input list into two halves, repeating the process on those halves, and finally merging the two sorted halves together. The algorithm first moves from top to bottom, dividing the list into smaller and smaller parts until only the separate elements remain.
Is merge sort incremental algorithm?
At last, all sub-arrays are merged to make it ‘n’ element size of the array….Tabular Representation:
Parameters | Merge Sort | Insertion Sort |
---|---|---|
Inplace Sorting | No | Yes |
Algorithm Paradigm | Divide and Conquer | Incremental Approach |
Why merge sort is not an in-place algorithm?
Because it copies more than a constant number of elements at some time, we say that merge sort does not work in place. By contrast, both selection sort and insertion sort do work in place, since they never make a copy of more than a constant number of array elements at any one time.
How to write a simple algorithm using pseudocode?
INPUT – indicates a user will be inputting something
How to implement merge sort?
How to Implement the Merge Sort Algorithm? It splits the input array in half, calls itself for each half, and then combines the two sorted parts. Merging two halves is done with the merge() method. Merge (array[], left, mid, right) is a crucial process that assumes array[left..mid] and array[mid+1..right] are both sorted sub-arrays and merges
How to program Merge sort?
Merge sort is used when the data structure doesn’t support random access,since it works with pure sequential access (forward iterators,rather than random access iterators).
How to implement merge sort without recursion?
Overview. In this tutorial,we’ll discuss how to implement the merge sort algorithm using an iterative algorithm.