How do you tuple in Python?
Table of Contents
How do you tuple in Python?
Creating a Tuple A tuple in Python can be created by enclosing all the comma-separated elements inside the parenthesis (). Elements of the tuple are immutable and ordered. It allows duplicate values and can have any number of elements. You can even create an empty tuple.
What is tuple in Python give example?
Tuple. Tuples are used to store multiple items in a single variable. Tuple is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Set, and Dictionary, all with different qualities and usage. A tuple is a collection which is ordered and unchangeable.
What is the basic idea of tuples?
A tuple is a collection of objects which ordered and immutable. Tuples are sequences, just like lists. The differences between tuples and lists are, the tuples cannot be changed unlike lists and tuples use parentheses, whereas lists use square brackets.

What are tuples good for in Python?
A tuple is useful for storing multiple values.. As you note a tuple is just like a list that is immutable – e.g. once created you cannot add/remove/swap elements. One benefit of being immutable is that because the tuple is fixed size it allows the run-time to perform certain optimizations.
How do I create a tuple list?
To convert the Python list to a tuple, use the tuple() function. The tuple() is a built-in function that passes the list as an argument and returns the tuple. The list elements will not change when it converts into a tuple.
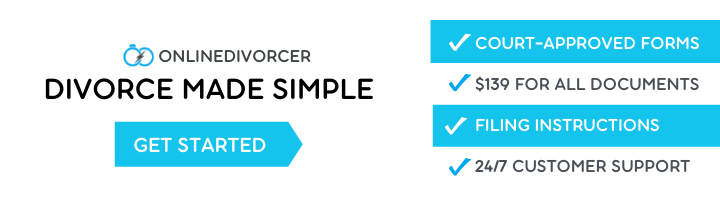
How do you write a tuple?
Creating tuples Tuples consist of values in parenthesis and separated by comma. Tuples can store values of different data types and duplicate values. We can also create tuples without using the parenthesis. A sequence of values separated by comma will create a tuple.
Why do you need tuples?
Tuples are more memory efficient than the lists. When it comes to the time efficiency, again tuples have a slight advantage over the lists especially when lookup to a value is considered. If you have data which is not meant to be changed in the first place, you should choose tuple data type over lists.
How do I add a tuple?
You can’t add elements to a tuple because of their immutable property. There’s no append() or extend() method for tuples, You can’t remove elements from a tuple, also because of their immutability.
How do you convert a string to a tuple?
When it is required to convert a string into a tuple, the ‘map’ method, the ‘tuple’ method, the ‘int’ method, and the ‘split’ method can be used. The map function applies a given function/operation to every item in an iterable (such as list, tuple). It returns a list as the result.
Why tuple is faster than list in Python?
Tuples are stored in a single block of memory. Tuples are immutable so, It doesn’t require extra space to store new objects. Lists are allocated in two blocks: the fixed one with all the Python object information and a variable-sized block for the data. It is the reason creating a tuple is faster than List.
What is the difference between a Python tuple and Python list?
The major difference between tuples and lists is that a list is mutable, whereas a tuple is immutable. This means that a list can be changed, but a tuple cannot.
When should you use tuples?
How tuples are stored in Python?
Tuple is stored in a single block of memory. Creating a tuple is faster than creating a list. Creating a list is slower because two memory blocks need to be accessed. An element in a tuple cannot be removed or replaced.
Is tuple important in Python?
In contrary, as tuples are immutable and fixed size, Python allocates just the minimum memory block required for the data. As a result, tuples are more memory efficient than the lists. As you can see from the output of the above code snippet, the memory required for the identical list and tuple objects is different.
Where are tuples used in real life?
You can use a Tuple to store the latitude and longitude of your home, because a tuple always has a predefined number of elements (in this specific example, two). The same Tuple type can be used to store the coordinates of other locations.
How tuples are stored in memory?
Tuples are stored in a single block of memory. Tuples are immutable so, It doesn’t require extra space to store new objects. Lists are allocated in two blocks: the fixed one with all the Python object information and a variable sized block for the data. It is the reason creating a tuple is faster than List.
How do you assign a value to a tuple in Python?
Once a tuple is created, you cannot change its values. Tuples are unchangeable, or immutable as it also is called. But there is a workaround. You can convert the tuple into a list, change the list, and convert the list back into a tuple.
How to create and use tuples in Python?
– Concatenation of Tuples – Nesting of Tuples – Repetition in Tuples. Try the above without a comma and check. – Immutable Tuples – Slicing in Tuples – Deleting a Tuple – Finding Length of a Tuple – Converting list to a Tuple. – Tuples in a loop – Using cmp (), max () , min () Note: max () and min () checks the based on ASCII values.
How do you use tuples in Python?
Defining. For the most part,tuples are defined in the exact same way as lists,just using parentheses instead of square brackets.
What is the point of a tuple in Python?
Tuples in Python can be used for defining, declaring and performing operations on a collection of values, and it is represented by the parenthesis (). These tuples have a limited set of pre-defined functions, and the values are of fixed length which cannot be updated or changed once it is created. In Python programming, the tuple functions are
What are the best Python tutorials?
Python Class by Google