How do you subtract two time strings in Python?
Table of Contents
How do you subtract two time strings in Python?
Use datetime. strptime() to Calculate Time Difference Between Two Time Strings in Python. The datatime class provides a user with a number of functions to deal with dates and times in Python. The strptime() function is used to parse a string value to represent time based on a given format.
How do I calculate time difference between hours in Python?
How to use Python’s datetime module
- import datetime. In order to calculate the time difference, you need to create two different timestamps.
- from datetime import date date1 = datetime.
- date1 = datetime.
- from datetime import time time1 = datetime.
- dt1 = datetime.
- dt2 = datetime.
- dt1 = datetime.
- today = datetime.
How do I calculate time difference between seconds in Python?
“python calculate time difference in seconds” Code Answer’s

- >>> import datetime.
- >>> a = datetime. datetime. now()
- >>> b = datetime. datetime. now()
- >>> c = b – a.
-
- >>> c.
- datetime. timedelta(0, 4, 316543)
- >>> c. days.
How do you subtract two datetime in Python?
Use datetime. datetime. combine() to subtract two datetime. time objects
- start_time = datetime. time(15, 30, 35)
- stop_time = datetime. time(13, 35, 50)
- date = datetime. date(1, 1, 1)
- datetime1 = datetime. datetime. combine(date, start_time)
- datetime2 = datetime. datetime. combine(date, stop_time)
How do you subtract time from datetime?
Steps to subtract N hours to datetime are as follows,
- Step 1: Get the current time in python using datetime.
- Step 2: Create an object of timedelta, to represent an interval of N hours.
- Step 3: Subtract the timedelta object from the datetime object pointing to current time.
How do you subtract hours and minutes in python?
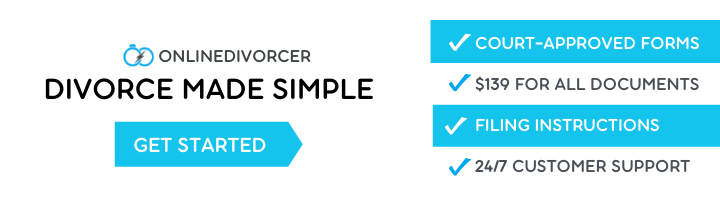
Subtract hours from given timestamp in python using relativedelta. In python, the dateutil module provides a class relativedelta, which represents an interval of time. The relativedelta class has all the attributes to represent a duration i.e. Year, Month, Day, Hours, Minutes, Seconds and Microseconds.
How do you calculate end time and start time in Python?
hour = int(input(“Starting time (hours): “)) mins = int(input(“Starting time (minutes): “)) dura = int(input(“Event duration (minutes): “)) print (“Event ending time: “,(hour + (mins + dura)//60)%24, “:”, (mins + dura%60)%60, sep=””)# Write your code here.
How does Python calculate time?
Use the below functions to measure the program’s execution time in Python:
- time. time() : Measure the the total time elapsed to execute the code in seconds.
- timeit.
- %timeit and %%timeit : command to get the execution time of a single line of code and multiple lines of code.
- datetime.
How does Python calculate runtime?
How do you subtract two datetime objects?
Subtraction of two datetime objects in Python:
- import datetime. d1 = datetime.datetime.now()
- d2 = datetime.datetime.now() x = d2-d1.
- print(type(x)) print(x)
How do you subtract date and time objects in Python?
How do you subtract 15 minutes from a time in Python?
“how to subtract minutes from time in python” Code Answer’s
- from datetime import datetime, timedelta.
-
- d = datetime. today() – timedelta(days=0, hours=0, minutes=0)
How do I subtract time from datetime?
The Subtract(DateTime) method determines the difference between two dates. To subtract a time interval from the current instance, call the Subtract(TimeSpan) method.
How do you calculate end time and start time?
For example, with start time of 9:00 AM and an end time of 5:00 PM, you can simply use this formula:
- =end-start =5:00PM-8:00AM =0.375-0.708=.333 // 8 hours.
- =1-start+end.
- =IF(end>start, end-start, 1-start+end)
- =MOD(end-start,1)
- 42614.4166666667 // date + time.
- =C5-B5 // end-start.
- [h]:mm.
How do I speed up my Python code?
A Few Ways to Speed Up Your Python Code
- Use proper data structure. Use of proper data structure has a significant effect on runtime.
- Decrease the use of for loop.
- Use list comprehension.
- Use multiple assignments.
- Do not use global variables.
- Use library function.
- Concatenate strings with join.
- Use generators.