How do you find the minimum of a function in C++?
Table of Contents
How do you find the minimum of a function in C++?
Program to find Maximum and minimum number in C++
- Assume the first element as max/min.
- Compare each element with the max/min.
- If, the element is greater than max or smaller then min, then, we change the value of max/min respectively.
- Then, output the value of max and/or min.
How do you find the maximum and minimum values of a function in C++?
int max=0, min=0; for(i=0;i>input; sum+=input; if(i==0){ max=input; min=input; } if(input>max) max=input; if(input
How do you find the minimum of two numbers in C++?

“how to find min of two numbers in c++” Code Answer
- int main()
- {
- int a = 5;
- int b = 7;
- cout << std::min(a, b) << “\n”;
- }
How do you find the minimum value in an array C++?
- Min or Minimum element can be found with the help of *min_element() function provided in STL.
- Max or Maximum element can be found with the help of *max_element() function provided in STL.
How do you find the smallest number in C++?
To find the smallest of elements in an integer array,
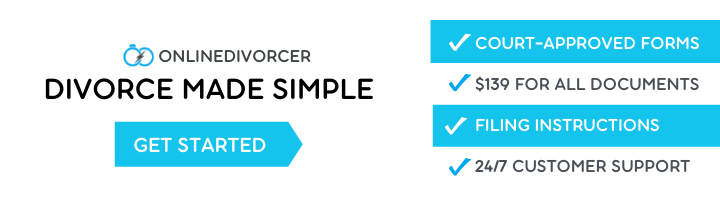
- Initialize smallest with first element of array.
- For each element in the array: Compare smallest with this element. If smallest is greater than this element, then update smallest with the element.
- smallest contains the smallest number in given integer array.
How do you find the minimum of 3 numbers in C++?
Show activity on this post.
- Simple Solution: int smallest(int x, int y, int z) { return std::min(std::min(x, y), z); }
- Better Solution (in terms of optimization): float smallest(int x, int y, int z) { return x < y? (
How do you find the smallest value in an array?
Java program to find the smallest number in an array
- Compare the first two elements of the array.
- If the first element is greater than the second swap them.
- Then, compare 2nd and 3rd elements if the second element is greater than the 3rd swap them.
- Repeat this till the end of the array.
How do you find the smallest number?
Steps to find the smallest number.
- Count the frequency of each digit in the number.
- If it is a non-negative number then. Place the smallest digit (except 0) at the left most of the required number.
- Else if it is a negative number then. Place the largest digit at the left most of the required number.
How do you find the minimum or maximum value of a function?
Finding max/min: There are two ways to find the absolute maximum/minimum value for f(x) = ax2 + bx + c: Put the quadratic in standard form f(x) = a(x − h)2 + k, and the absolute maximum/minimum value is k and it occurs at x = h. If a > 0, then the parabola opens up, and it is a minimum functional value of f.
How do you find the minimum value of a set of data?
The minimum is the first number listed as it is the lowest, and the maximum is the last number listed because it is the highest.
How do you find the minimum value of three elements?
Algorithm
- Start.
- Take three numbers in a, b, c.
- Check if a is less than b and a is less than c.
- If above condition is true, a is smallest and go to step 7, else go to step 5.
- Check if b is less than c.
- If above condition is true, b is the smallest, else c is the smallest.
- Stop.
How do you find the minimum value in an array in CPP?
What values are passed by reference?
Passing a variable To make it short: pass by value means the actual value is passed on. Pass by reference means a number (called an address) is passed on which defines where the value is stored.