How do you do a linear search in an array?
Table of Contents
How do you do a linear search in an array?
A simple approach to implement a linear search is
- Begin with the leftmost element of arr[] and one by one compare x with each element.
- If x matches with an element then return the index.
- If x does not match with any of the elements then return -1.
Can linear search be done using arrays?
Linear or Sequential Search This algorithm works by sequentially iterating through the whole array or list from one end until the target element is found. If the element is found, it returns its index, else -1.
How do you write a linear search method in Java?
Linear search is used to search a key element from multiple elements….Linear Search in Java

- Step 1: Traverse the array.
- Step 2: Match the key element with array element.
- Step 3: If key element is found, return the index position of the array element.
- Step 4: If key element is not found, return -1.
What is linear search algorithm with example?
Example of Linear Search Algorithm Step 1: The searched element 39 is compared to the first element of an array, which is 13. The match is not found, you now move on to the next element and try to implement a comparison. Step 2: Now, search element 39 is compared to the second element of an array, 9.
What are the search algorithms Java?
Search Algorithms in Java
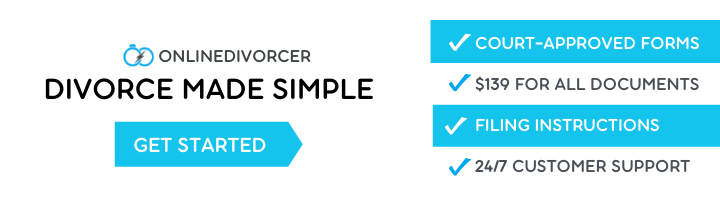
- Linear Search.
- Binary Search.
- Knuth Morris Pratt Pattern Search.
- Jump Search.
- Interpolation Search.
- Exponential Search.
- Fibonacci Search.
- Java Collections API.
When would you go for linear search in an array and why?
Linear search is usually very simple to implement, and is practical when the list has only a few elements, or when performing a single search in an un-ordered list. When many values have to be searched in the same list, it often pays to pre-process the list in order to use a faster method.
How do you write a linear search algorithm?
Algorithm
- Step 1: Select the first element as the current element.
- Step 2: Compare the current element with the target element.
- Step 3: If there is a next element, then set current element to next element and go to Step 2.
- Step 4: Target element not found.
- Step 5: Target element found and return location.
What is linear search in Java?
Linear search is a very simple search algorithm. In this type of search, a sequential search is done for all items one by one. Every item is checked and if a match is found then that particular item is returned, otherwise the search continues till the end of the data collection.
What are linear arrays with example?
LINEAR ARRAYS is a graphic organizer that helps students visualize gradations of meaning between two related words. It is used before or after reading to examine subtle distinctions in words. This strategy develops students’ word consciousness, illustrating how each word has a specific meaning.
What is the use of linear search algorithm?
In computer science, a linear search or sequential search is a method for finding an element within a list. It sequentially checks each element of the list until a match is found or the whole list has been searched.
Where is linear search algorithm used?
It is widely used to search an element from the unordered list, i.e., the list in which items are not sorted. The worst-case time complexity of linear search is O(n). First, we have to traverse the array elements using a for loop. If the element matches, then return the index of the corresponding array element.
What is search in array?
One of the basic operations to be performed on an array is searching. Searching an array means to find a particular element in the array. The search can be used to return the position of the element or check if it exists in the array.
What is linear array in Java?
A linear array, is a list of finite numbers of elements stored in the memory. In a linear array, we can store only homogeneous data elements. Elements of the array form a sequence or linear list, that can have the same type of data. Each element of the array, is referred by an index set.
What is an array in algorithm?
Array is a container which can hold a fix number of items and these items should be of the same type. Most of the data structures make use of arrays to implement their algorithms. Following are the important terms to understand the concept of Array. Element − Each item stored in an array is called an element.
What is complexity of linear search algorithm?
The complexity of linear search is therefore O(n). If the element to be searched lived on the the first memory block then the complexity would be: O(1). The code for a linear search function in JavaScript is shown below. This function returns the position of the item we are looking for in the array.
How to perform linear and binary search in Java?
Linear Search in Java. Linear search is used to search a key element from multiple elements. Linear search is less used today because it is slower than binary search and hashing. Algorithm: Step 1: Traverse the array. Step 2: Match the key element with array element. Step 3: If key element is found, return the index position of the array element.
What is the best searching algorithm?
– Binary search has a restriction that you should be able to divide the array into 2 parts – So either you should have sorted array – or t t t t t t t f f f f f f f, some of the elements should evaluate to true and some false for a condition
What is an example of a linear search?
Linear search can be applied to both single-dimensional and multi-dimensional arrays.
What is linear search?
A linear search is the simplest method of searching a data set. Starting at the beginning of the data set, each item of data is examined until a match is made. Once the item is found, the search ends.