How do you check if array does not include JavaScript?
Table of Contents
How do you check if array does not include JavaScript?
To check if an array doesn’t include a value, use the logical NOT (!) operator to negate the call to the includes() method. The NOT (!) operator returns false when called on a true value and vice versa.
Does array contain JavaScript?
The array includes() is a built-in JavaScript function that checks whether an array contains a specified element. There is no built-in array contains() function in JavaScript.
How do you check if an array contains an object JavaScript?
Using includes() Method: If array contains an object/element can be determined by using includes() method. This method returns true if the array contains the object/element else return false. Example: html.

How do you check a string is present in array or not in JavaScript?
To check if a JavaScript Array contains a substring:
- Call the Array. findIndex method, passing it a function.
- The function should return true if the substring is contained in the array element.
- If the conditional check succeeds, Array. findIndex returns the index of the array element that matches the substring.
How do you know if something is not in an array?
Answer: Use the Array. isArray() Method You can use the JavaScript Array. isArray() method to check whether an object (or a variable) is an array or not. This method returns true if the value is an array; otherwise returns false .
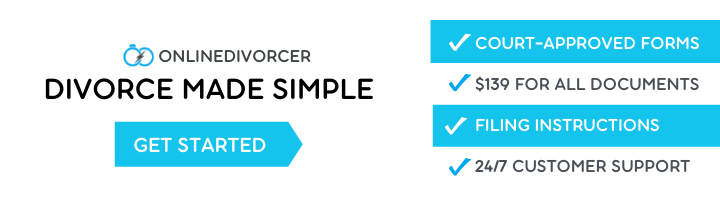
How do you check if an array contains an item?
The simplest and fastest way to check if an item is present in an array is by using the Array. indexOf() method. This method searches the array for the given item and returns its index. If no item is found, it returns -1.
How do you check if an array is undefined?
Use the includes() method to check if an array contains undefined values, e.g. arr. includes(undefined) . The includes method will return true if the array contains at least one undefined value and false otherwise.
How do you check if an array of objects is empty in JavaScript?
Method 1: Using Array. isArray() method and array. length property: The array can be check if it is actually an array and it exists by the Array.
How do you check an object is empty or not in JavaScript?
Check if an Object is Empty in JavaScript #
- Pass the object to the Object. keys method to get an array of all the keys of the object.
- Access the length property on the array.
- Check if the length of keys is equal to 0 , if it is, then the object is empty.
When a array is undefined in JavaScript?
How do I check if an array contains an empty object?
Use the Object. entries() function. It returns an array containing the object’s enumerable properties. If it returns an empty array, it means the object does not have any enumerable property, which in turn means it is empty.
How do you check if an array of objects is empty?
length property. If the length of the object is 0, then the array is considered to be empty and the function will return TRUE. Else the array is not empty and the function will return False.
How do you check if an element in an array is empty JavaScript?
To check if an array is empty or not, you can use the . length property. The length property sets or returns the number of elements in an array. By knowing the number of elements in the array, you can tell if it is empty or not.
How check object is empty or not in JavaScript?
How do you check if an element in an array is empty?
To check if an array is null, use equal to operator and check if array is equal to the value null. In the following example, we will initialize an integer array with null. And then use equal to comparison operator in an If Else statement to check if array is null. The array is empty.
How do I check if an array contains an empty string?
To check if an array contains an empty string, call the includes() method passing it an empty string – includes(”) . The includes method returns true if the provided value is contained in the array and false otherwise. Copied!