How do I display a date without time in Python?
Table of Contents
How do I display a date without time in Python?
To remove the time from a datetime object in Python, convert the datetime to a date using date(). You can also use strftime() to create a string from a datetime object without the time.
How do you set a specific time in Python?
“python set a specific datetime” Code Answer
- from datetime import datetime.
-
- custom_date_time = datetime(2021, 7, 23, 17, 30, 29, 431717)
- print(custom_date_time)
-
- # Output:
- # 2021-07-23 17:30:29.431717.
How do I get only the date in Python?
Convert datetime Object to Date Only String in Python (Example)

- 1) Import datetime Module & Create Example Data.
- 2) Example 1: Extract Only Date from datetime Object Using strftime() Function.
- 3) Example 2: Extract Only Date from datetime Object Using %s Operator.
How do I get the current date and time in Python?
today() method to get the current local date. By the way, date. today() returns a date object, which is assigned to the today variable in the above program. Now, you can use the strftime() method to create a string representing date in different formats.
How do you display time in Python?
How to Get the Current Date and Time in Python
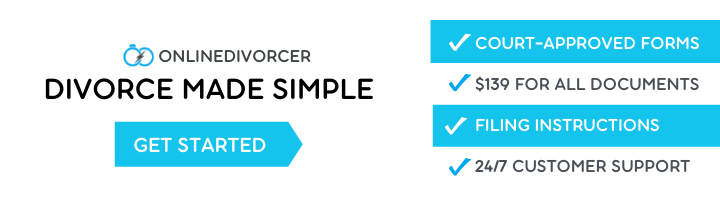
- Command line / terminal window access.
- Options for datetime formating.
- Use strftime() to display Time and Date.
- Save and close the file.
- To display the time in a 12-hour format, edit the file to: import time print (time.strftime(“%I:%M:%S”))
What is %z in datetime Python?
List of the Date format codes:
Directive | Meaning | Example |
---|---|---|
%Z | Time zone name (empty string if the object is naive). | (empty), UTC, EST, CST |
%j | Day of the year as a zero-padded decimal number. | 001, 002, …, 366 |
How do I run a code at a specific time?
You can use perform(_:with:afterDelay:) to run a method after a certain number of seconds have passed, but if you want to run code at a specific time – say at exactly 4pm – then you should use Timer instead.
How do I show time in Python?
How do I print UTC time in Python?
datetime. now() to get the current date and time. Then use tzinfo class to convert our datetime to UTC. Lastly, use the timestamp() to convert the datetime object, in UTC, to get the UTC timestamp.
How do I set UTC time zone in Python?
We’ll use the following attributes and methods of the pytz module to work with timezone in Python.
- pytz.utc : Get the standard UTC timezone.
- pytz.timezone(‘region’) : Create the timezone object of a particular region.
- pytz.astimezone() : Convert the time of a particular time zone into another time zone.
How do you record time in Python?
“how to record execution time in python” Code Answer
- import time.
-
- start = time. time()
- print(“hello”)
- end = time. time()
- print(end – start)
Is time time () in seconds Python?
Pythom time method time() returns the time as a floating point number expressed in seconds since the epoch, in UTC.
How do I run a function at a specific time in python?
Schedule lets you run Python functions (or any other callable) periodically at pre-determined intervals using a simple, human-friendly syntax. Schedule Library is used to schedule a task at a particular time every day or a particular day of a week. We can also set time in 24 hours format that when a task should run.
How do I use python sched?
The process of scheduling events through sched module requires us to follow a list of simple steps.
- Create a scheduler instance using scheduler() method of sched module.
- Create events using one of the enter() or enterabs() method of scheduler instance.
- Call run() method on scheduler instance to start executing events.
How do I convert datetime to date in python?
How to convert a string to a date in Python
- from datetime import datetime.
-
- date_time_str = ’18/09/19 01:55:19′
-
- date_time_obj = datetime. strptime(date_time_str, ‘%d/%m/%y %H:%M:%S’)
-
-
- print (“The type of the date is now”, type(date_time_obj))
How do I set UTC time in Python?
You can use the datetime module to convert a datetime to a UTC timestamp in Python. If you already have the datetime object in UTC, you can the timestamp() to get a UTC timestamp. This function returns the time since epoch for that datetime object.
How do I get absolute time in Python?
Get Current Time in Python Use the time. time() function to get the current time in seconds since the epoch as a floating-point number. This method returns the current timestamp in a floating-point number that represents the number of seconds since Jan 1, 1970, 00:00:00.