Can you cast an int to a char C++?
Table of Contents
Can you cast an int to a char C++?
Convert int to a char in C++ A better, safer option to cast an integer to a char is using static_cast , as shown below. C++ also offers three other casting operators – dynamic_cast , reinterpret_cast , and const_cast (Read more here). That’s all about converting an int to a char in C++.
How do I cast an int to a char?
Example 1: Java Program to Convert int to char char a = (char)num1; Here, we are using typecasting to covert an int type variable into the char type variable. To learn more, visit Java Typecasting. Note that the int values are treated as ASCII values.
Can you cast int to string C++?
Conversion of an integer into a string by using to_string() method. The to_string() method accepts a single integer and converts the integer value or other data type value into a string.
What happens when casting int to char?

We can convert int to char in java using typecasting. To convert higher data type into lower, we need to perform typecasting. Here, the ASCII character of integer value will be stored in the char variable. To get the actual value in char variable, you can add ‘0’ with int variable.
How do I convert one data type to another in C++?
In C++, it can be done by two ways:
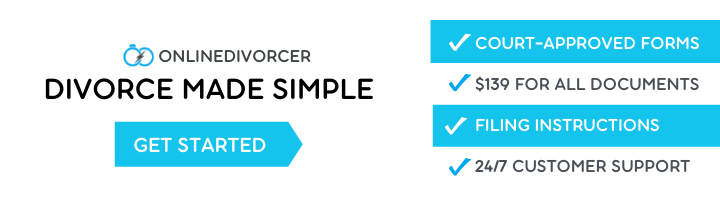
- Converting by assignment: This is done by explicitly defining the required type in front of the expression in parenthesis.
- Conversion using Cast operator: A Cast operator is an unary operator which forces one data type to be converted into another data type.
Can you cast an int to a char c?
Casting int to char is done simply by assigning with the type in parenthesis: int i = 65535; char c = (char)i; Note: I thought that you might be losing data (as in the example), because the type sizes are different.
What is itoa () in C?
itoa () function is used to convert int data type to string data type in C language.
How do you cast a variable in C++?
Typecasting is making a variable of one type, such as an int, act like another type, a char, for one single operation. To typecast something, simply put the type of variable you want the actual variable to act as inside parentheses in front of the actual variable. (char)a will make ‘a’ function as a char.
What is casting operator in C++?
A cast is a special operator that forces one data type to be converted into another. As an operator, a cast is unary and has the same precedence as any other unary operator. const_cast (expr) − The const_cast operator is used to explicitly override const and/or volatile in a cast.
Can char take integer values?
The chr() function takes an integer value in a range.
What is stoi C++?
In C++, the stoi() function converts a string to an integer value. The function is shorthand for “string to integer,” and C++ programmers use it to parse integers out of strings.
How do you type cast in C++?
How do I use itoa in C++?
Converts an integer value to a null-terminated string using the specified base and stores the result in the array given by str parameter. If base is 10 and value is negative, the resulting string is preceded with a minus sign (-). With any other base, value is always considered unsigned.
How do I use itoa in C ++?
char * itoa ( int value, char * str, int base ); “stdlib. h” header file supports all the type casting functions in C language. But, it is a non standard function….
Typecast function | Description |
---|---|
atol() | atol( ) function converts string to long |
itoa() | itoa( ) function converts int to string |