Can you access HashMap by index?
Table of Contents
Can you access HashMap by index?
In the ArrayList chapter, you learned that Arrays store items as an ordered collection, and you have to access them with an index number ( int type). A HashMap however, store items in “key/value” pairs, and you can access them by an index of another type (e.g. a String ).
Can we get index in HashMap in Java?
The HashMap has no defined ordering of keys. Show activity on this post. Show activity on this post. You can’t – a set is unordered, so there’s no index provided.
How do you find the index value of a map?
Getting the index in map() We can get the index of the current iteration using the 2nd parameter of the map() function. const lst = [1, 2, 3]; lst. map((elem, index) => { console. log(`Element: ${elem}`); console.

How do I iterate over a HashMap?
Example 1: Iterate through HashMap using the forEach loop
- languages.entrySet() – returns the set view of all the entries.
- languages.keySet() – returns the set view of all the keys.
- languages.values() – returns the set view of all the values.
How do you find the index of a Map?
To find an index of the current iterable inside the JavaScript map() function, you have to use the callback function’s second parameter. An index used inside the JavaScript map() method is to state the position of each item in an array, but it doesn’t modify the original array.
How do I find the index of an element on a Map?
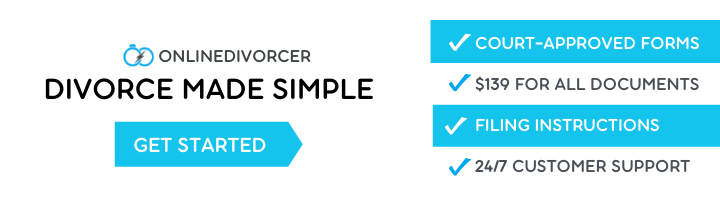
Index inside map() Function The index is used inside map() method to state the position of each element in an array, but it doesn’t change the original array. Syntax: array. map(function(currentelement, index, arrayobj) { // Returns the new value instead of the item });
How do you iterate through a HashMap?
How do I retrieve a value from a Map?
Generally, To get all keys and values from the map, you have to follow the sequence in the following order:
- Convert Hashmap to MapSet to get set of entries in Map with entryset() method.: Set st = map.
- Get the iterator of this set: Iterator it = st.
- Get Map.
- use getKey() and getValue() methods of the Map.
How do I get the value of a Map for a specific key?
HashMap get() Method in Java get() method of HashMap class is used to retrieve or fetch the value mapped by a particular key mentioned in the parameter. It returns NULL when the map contains no such mapping for the key.
How do I find the value of a key on a Map?
How do you check if a HashMap contains a value?
HashMap containsValue() Method in Java HashMap. containsValue() method is used to check whether a particular value is being mapped by a single or more than one key in the HashMap. It takes the Value as a parameter and returns True if that value is mapped by any of the key in the map.
How do you find the index of an element in a list Dart?
In Dart, the List class has 4 methods that can help you find the index of a specific element in a list: indexOf: Returns the first index of the first element in the list that equals to a given element. Returns -1 if nothing found. indexWhere: Returns the first index in the list that satisfies the given conditions.
How do I find the value of a key on a map?
What is the best way to iterate HashMap in java?
There is a numerous number of ways to iterate over HashMap of which 5 are listed as below:
- Iterate through a HashMap EntrySet using Iterators.
- Iterate through HashMap KeySet using Iterator.
- Iterate HashMap using for-each loop.
- Iterating through a HashMap using Lambda Expressions.
- Loop through a HashMap using Stream API.