Can ArrayList be converted to String?
Table of Contents
Can ArrayList be converted to String?
To convert the contents of an ArrayList to a String, create a StringBuffer object append the contents of the ArrayList to it, finally convert the StringBuffer object to String using the toString() method.
How do I turn a List of objects into strings?
List lst String arr = lst. toString();
How do you add an ArrayList to a String array in Java?
Approach:

- Get the ArrayList of String.
- Convert ArrayList to Object array using toArray() method.
- Iterate and convert each element to the desired type using typecasting. Here it is converted to String type and added to the string array.
- Print the string array.
How will you convert an ArrayList to arrays?
Following methods can be used for converting ArrayList to Array:
- Method 1: Using Object[] toArray() method.
- Method 2: Using T[] toArray(T[] a)
- Method 3: Manual method to convert ArrayList using get() method.
- Method 4: Using streams API of collections in java 8 to convert to array of primitive int type.
Can we convert list to String array in Java?
The ArrayList can be easily converted using Spring’s StringUtils class. In the Java collection framework, we can convert our data structure from ArrayList to String Array using the following methods: Using ArrayList. get() method.
Can we convert list to String in Java?
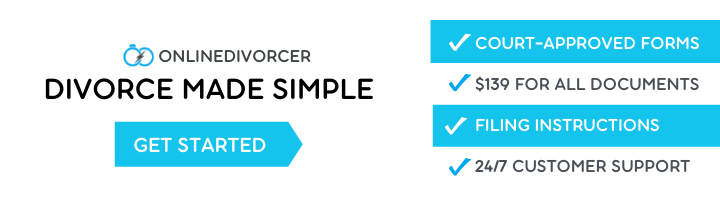
We use the toString() method of the list to convert the list into a string.
How do I convert a list of elements to a string in Java?
2. Using Java 8 and above
- import java. util. Arrays;
- import java. util. List;
- class Main.
- public static void main(String[] args)
- {
- List list = Arrays. asList(“A”, “B”, “C”);
- String delim = “-“;
- String res = String. join(delim, list);
How do you put an array into a String?
Using StringBuffer
- Create an empty String Buffer object.
- Traverse through the elements of the String array using loop.
- In the loop, append each element of the array to the StringBuffer object using the append() method.
- Finally convert the StringBuffer object to string using the toString() method.
How do I turn a list into an array?
Java program to convert a list to an array
- Create a List object.
- Add elements to it.
- Create an empty array with size of the created ArrayList.
- Convert the list to an array using the toArray() method, bypassing the above-created array as an argument to it.
- Print the contents of the array.
How do you change an object to an array in Java?
Following are the steps:
- Convert the specified object array to a sequential Stream.
- Use Stream. map() to convert every object in the stream to their integer representation.
- Use the toArray() method to accumulate the stream elements into a new integer array.
How do I turn a List into an array?
How do you convert a List to an array in Java?
How do you convert a string to an ArrayList string in Java?
Approach:
- Splitting the string by using the Java split() method and storing the substrings into an array.
- Creating an ArrayList while passing the substring reference to it using Arrays. asList() method.
How do you convert a list to an array in Java?
What is toString method in Java?
Java – toString() Method The method is used to get a String object representing the value of the Number Object. If the method takes a primitive data type as an argument, then the String object representing the primitive data type value is returned.
How do I combine array elements into strings?
The arr. join() method is used to join the elements of an array into a string. The elements of the string will be separated by a specified separator and its default value is a comma(, ).
How do you join an array to a string in Java?
To join elements of given string array strArray with a delimiter string delimiter , use String. join() method. Call String. join() method and pass the delimiter string delimiter followed by the string array strArray .
How do you create an array from a list in Java?
Your task is to convert the given array into a list in Java….Algorithm:
- Get the Array to be converted.
- Convert the array to Stream.
- Convert the Stream to List using Collectors. toList()
- Collect the formed list using the collect() method.
- Return the formed List.
What is toList () in Python?
tolist(), used to convert the data elements of an array into a list. This function returns the array as an a. ndim- levels deep nested list of Python scalars. In simple words, this function returns a copy of the array elements as a Python list.