What is the output of Dijkstra algorithm?
Table of Contents
What is the output of Dijkstra algorithm?
Dijkstra’s original algorithm found the shortest path between two given nodes, but a more common variant fixes a single node as the “source” node and finds shortest paths from the source to all other nodes in the graph, producing a shortest-path tree.
What is the problem with Dijkstra algorithm?
Since Dijkstra follows a Greedy Approach, once a node is marked as visited it cannot be reconsidered even if there is another path with less cost or distance. This issue arises only if there exists a negative weight or edge in the graph.
What is the input and output for Dijkstra’s algorithm?
1.9 Input and output data of the algorithm Input data: weighted graph (V, E, W) (n nodes v_i and m arcs e_j = (v^{(1)}_{j}, v^{(2)}_{j}) with weights f_j), source node u. Size of input data: O(m + n). Output data (possible variants):

What is the formula for Dijkstra’s algorithm?
D5 = min {D5, D6 +R6,5} = min {7, 5+1} = 6. The values circled are the sought minimal distances from node 1 to other nodes. An oriented weighted graph is given (V, E) with n nodes V and arcs E, which does not have negative weights. Find the minimal distances from node numbered p to all the other nodes of the graph.
What is the complexity of Dijkstra algorithm represented in matrix?
The complexity of Dijkstra’s shortest path algorithm is O(E log V) as the graph is represented using adjacency list.
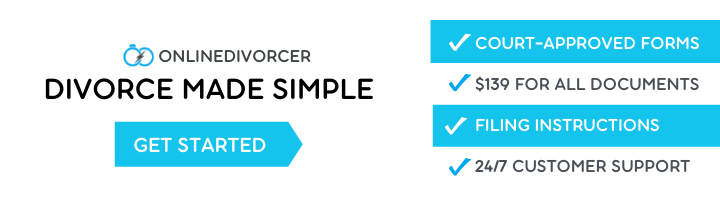
Why did Dijkstra fail negative weights?
Since Dijkstra’s goal is to find the optimal path (not just any path), it, by definition, cannot work with negative weights, since it cannot find the optimal path. Dijkstra will actually not loop, since it keeps a list of nodes that it has visited. But it will not find a perfect path, but instead just any path.
How do you solve Dijkstra’s shortest path algorithm?
We step through Dijkstra’s algorithm on the graph used in the algorithm above:
- Initialize distances according to the algorithm.
- Pick first node and calculate distances to adjacent nodes.
- Pick next node with minimal distance; repeat adjacent node distance calculations.
- Final result of shortest-path tree.
How do you calculate the efficiency of Dijkstra’s algorithm?
This is step-by-step for one way to check the efficiency.
- Implement Dijkstras algorithm in a programming language of your preference.
- Find an existing implementation or implement a second algorithm.
- Run the algorithms of the same input data on the same computer.
- a Verify that the shortest path actually was found.
What is the complexity of Dijkstra algorithm represented in Matrix?
What is the complexity of Dijkstra’s algorithm operates on graph has 5 nodes and 7 edges?
Time complexity of Dijkstra’s algorithm is O ( V 2 ) O(V^2) O(V2) where V is the number of verices in the graph. It can be explained as below: First thing we need to do is find the unvisited vertex with the smallest path. For that we require O ( V ) O(V) O(V) time as we need check all the vertices.
How does Dijkstra’s algorithm calculate efficiency?
Can Dijkstra’s algorithm handle negative weight edges Why or why not?
Since Dijkstra’s goal is to find the optimal path (not just any path), it, by definition, cannot work with negative weights, since it cannot find the optimal path.
Is Dijkstra algorithm static or dynamic?
Another way to solve this problem is to make the Dijkstra algorithm dynamic. The Static Dijkstra algorithm is an iterative algorithm which is used to find the shortest path from a specific vertex of the graph called as source vertex to all the other vertices of the graph (Dijkstra, 1959).
Is Dijkstra’s algorithm optimal?
Dijkstra’s algorithm is used for graph searches. It is optimal, meaning it will find the single shortest path. It is uninformed, meaning it does not need to know the target node before hand. In fact it finds the shortest path from every node to the node of origin.