What is data Map in JavaScript?
Table of Contents
What is data Map in JavaScript?
A Map is a collection of key🔑 — value pairs, similar to an object. It stores the key🔑 — value pairs in the insertion order. We can create a Map by passing an iterable object whose elements are in the form of key🔑 — value (Eg.
Is there a Map in JavaScript?
JavaScript 2015 (ES6) introduced a feature called Map. Not to be confused with the . map() array method, the built-in Map object is another way to structure your data. Maps are collections of distinct and ordered key-value pairs.
How do you Map an object in JavaScript?
To convert an object to a Map , call the Object. entries() method to get an array of key-value pairs and pass the result to the Map() constructor, e.g. const map = new Map(Object. entries(obj)) . The new Map will contain all of the object’s key-value pairs.
How do I Map an array in JavaScript?

The map() method in JavaScript creates an array by calling a specific function on each element present in the parent array. It is a non-mutating method. Generally map() method is used to iterate over an array and calling function on every element of array.
How do I Map data from API?
Getting Started With API Data Mapping
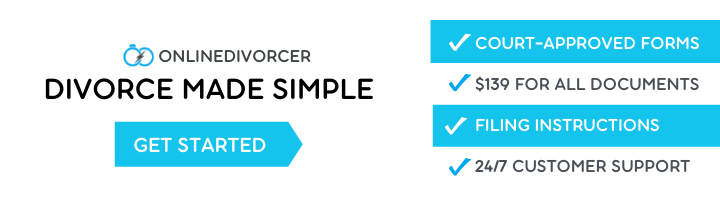
- Step 1: Data Discovery.
- Step 2: Define a Default Map.
- Step 3: Map Custom Data From Each Instance.
- Step 4: Make Your “Default” A Guideline, Not a Requirement.
- Step 5: Leverage Self-Service Data Mapping Tools.
What is Map data structure?
• A Map is an abstract data structure (ADT) • it stores key-value (k,v) pairs. • there cannot be duplicate keys. • Maps are useful in situations where a key can be viewed as a unique identifier for the object. • the key is used to decide where to store the object in the structure.
Why is a Map () important in JavaScript?
JavaScript Map creates a new array, which contains the results from the iteration over the elements of the array and calls the provided function once for each element in order. It’s an important data structure that has many essential uses.
What is map data structure?
What is map object in JavaScript?
The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
How do you create a map from an array?
To convert an array of objects to a Map , call the map() method on the array and on each iteration return an array containing the key and value. Then pass the array of key-value pairs to the Map() constructor to create the Map object.
How do I iterate a map in JavaScript?
Iterate through a Map using JavaScript # Use the forEach() method to iterate over a Map object. The forEach method takes a function that gets invoked for each key/value pair in the Map , in insertion order. The function gets passed the value, key and the Map object on each iteration.
How is map data stored?
Content of a map database Maps are stored as graphs, or two dimensional arrays of objects with attributes of location and category, where some common categories include parks, roads, cities, and the like. A map database represents a road network along with associated features.
What is use of map data structure?
A Map is a type of fast key lookup data structure that offers a flexible means of indexing into its individual elements.
What is difference between JSON and map?
A JSONObject is an unordered collection of name/value pairs whereas Map is an object that maps keys to values. A Map cannot contain duplicate keys and each key can map to at most one value. We need to use the JSON-lib library for serializing and de-serializing a Map in JSON format.
Is JavaScript map better than object?
Although Map tends to have more advantages over objects, at the end the day it depends on the kind of data being used and the operation needs to be performed. However, of all the advantages of map over object, map cannot replace object in JavaScript because Object is much more than a hash table.
Why map is introduced in JavaScript?
Maps are a new data structure in JavaScript that allows you to create collections of key-value pairs. They were introduced with ES6 (also called ES2015) along with Sets in JavaScript. A map object can store both objects and primitives as keys and values.
Is JavaScript map a Hashmap?
javascript object is a real hashmap on its implementation, so the complexity on search is O(1), but there is no dedicated hashcode() function for javascript strings, it is implemented internally by javascript engine (V8, SpiderMonkey, JScript. dll, etc…)
How do I create a map in JavaScript?
new Map () – creates the map. map.set (key, value) – stores the value by the key. map.get (key) – returns the value by the key, undefined if key doesn’t exist in map. map.has (key) – returns true if the key exists, false otherwise. map.delete (key) – removes the value by the key.
How to find the size of map in JavaScript?
Map is a collection of elements where each element is stored as a Key, value pair. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair in the same order as inserted. Map.prototype.size – It returns the number of elements or the key-value pairs in the map.
How to use map JavaScript?
let map = new Map ( [iterable]); Code language: JavaScript (javascript) The Map () accepts an optional iterable object whose elements are key-value pairs. Useful JavaScript Map methods clear () – removes all elements from the map object. delete (key) – removes an element specified by the key.
How to check if JavaScript map has an object key?
Syntax. The String name or Symbol of the property to test.