What does expression must have class type mean?
Table of Contents
What does expression must have class type mean?
The “expression must have class type” is an error that is thrown when the . operator, which is typically used to access an object’s fields and methods, is used on pointers to objects.
Can I use this pointer in constructor c++?
Some people feel you should not use the this pointer in a constructor because the object is not fully formed yet. However you can use this in the constructor (in the { body } and even in the initialization list) if you are careful.
What is a pointer to object type in C++?
In C++, a pointer holds the address of an object stored in memory. The pointer then simply “points” to the object. The type of the object must correspond with the type of the pointer. type *name; // points to a value of the specified type.

What does expression must be a modifiable lvalue mean?
expression must be a modifiable lvalue An lvalue is value that is allowed to be on the Left hand side of an assignment statement. For example in the following statement, the variable Value is the lvalue as it is on the left hand side of the statement and is having its value modified to be 24. 1.
What is a class constructor in C++?
A class constructor is a special member function of a class that is executed whenever we create new objects of that class. A constructor will have exact same name as the class and it does not have any return type at all, not even void.
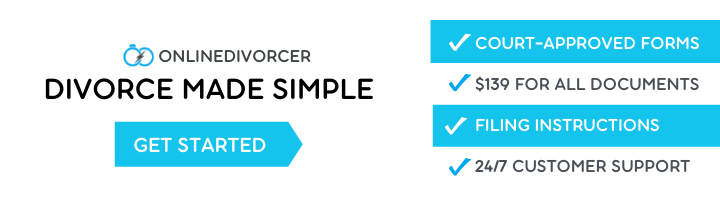
Can a class have multiple constructors?
There can be multiple constructors in a class. However, the parameter list of the constructors should not be same. This is known as constructor overloading.
Can you have a pointer to a class?
A pointer to a C++ class is done exactly the same way as a pointer to a structure and to access members of a pointer to a class you use the member access operator -> operator, just as you do with pointers to structures. Also as with all pointers, you must initialize the pointer before using it.
Can a pointer point to a class?
As we just saw pointer to class, a pointer can also point to an an array of class. That is it can be used to access the elements of an array of type class.
What are Lvalues and Rvalues in C++?
Lvalues and rvalues are fundamental to C++ expressions. Put simply, an lvalue is an object reference and an rvalue is a value. The difference between lvalues and rvalues plays a role in the writing and understanding of expressions.
What is lvalue required as left operand of assignment?
lvalue required as left operand of assignment. lvalue means an assignable value (variable), and in assignment the left value to the = has to be lvalue (pretty clear). Both function results and constants are not assignable ( rvalue s), so they are rvalue s.
Can a class have only one constructor?
According to MDN (my favorite go-to resource): Having more than one occurrence of a constructor method in a class will throw a SyntaxError error.. So yes, a class can only have one constructor.
Can a class be a pointer C++?
A pointer to a C++ class is done exactly the same way as a pointer to a structure and to access members of a pointer to a class you use the member access operator -> operator, just as you do with pointers to structures.
What is a pointer to class type?
A class pointer is a pointer variable that stores address of an object of a class. As shown in the above diagram we have a class Rectangle with 2 data members and 1 member function. We have also created an object of that class named var1.
Which type of class Cannot be created?
of abstract class
Explanation: Instance of abstract class can’t be created as it will not have any constructor of its own, hence while creating an instance of class, it can’t initialize the object members. Actually the class inheriting the abstract class can have its instance because it will have implementation of all members.
What are Lvalues and Rvalues?
An lvalue (locator value) represents an object that occupies some identifiable location in memory (i.e. has an address). rvalues are defined by exclusion. Every expression is either an lvalue or an rvalue, so, an rvalue is an expression that does not represent an object occupying some identifiable location in memory.
Are rvalue references Lvalues?
“l-value” refers to a memory location that identifies an object. “r-value” refers to the data value that is stored at some address in memory. References in C++ are nothing but the alternative to the already existing variable.
What is lvalue required?
This error occurs when we put constants on left hand side of = operator and variables on right hand side of it. Example: #include void main()