How to find a key in a map in C++?
Table of Contents
How to find a key in a map in C++?
Find a Key in C++ Map
- Use std::map::find Method to Find a Key in a Map.
- Use std::map::count Method to Find a Key in a Map.
- Use std::map::contains Method to Find a Key in a Map.
What does map find() do?
C++ map find() function is used to find an element with the given key value k. If it finds the element then it returns an iterator pointing to the element. Otherwise, it returns an iterator pointing to the end of the map, i.e., map::end().
How does a map store values?
Maps are associative containers that store elements in a combination of key values and mapped values that follow a specific order. No two mapped values can have the same key values. In C++, maps store the key values in ascending order by default.

What does find return in map?
map find() function in C++ STL Return Value: The function returns an iterator or a constant iterator which refers to the position where the key is present in the map. If the key is not present in the map container, it returns an iterator or a constant iterator which refers to map.
Can map have duplicate keys?
Duplicate keys are not allowed in a Map. Basically, Map Interface has two implementation classes HashMap and TreeMap the main difference is TreeMap maintains an order of the objects but HashMap will not.
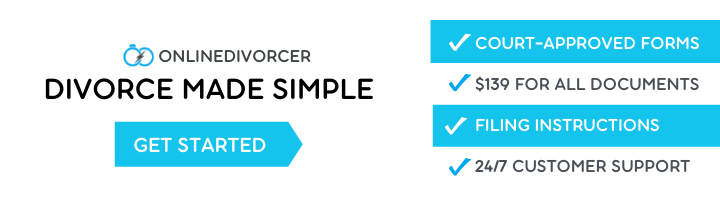
How do you find the value of a map?
Search by value in a Map in C++ Given a set of N pairs as a (key, value) pairs in a map and an integer K, the task is to find all the keys mapped to the give value K. If there is no key value mapped to K then print “-1”. Explanation: The 3 key value that is mapped to value 3 are 1, 2, 10.
Can a HashMap store objects?
Internal working. All instances of Entry class are stored in an array declard as ‘transient Entry[] table’ . For each key-value to be stored in HashMap, a hash value is calculated using the key’s hash code. This hash value is used to calculate the index in the array for storing Entry object.
Does .map need a return?
map() method allows you to loop over every element in an array and modify or add to it and then return a different element to take that elements place. However . map() does not change the original array. It will always return a new array.
How do you find contains on a map?
java. util. HashMap. containsKey() Method
- Description. The containsKey(Object key) method is used to check if this map contains a mapping for the specified key.
- Declaration. Following is the declaration for java.
- Parameters. key − This is the key whose presence in this map is to be tested.
- Return Value.
- Exception.
- Example.
How do you check if a map contains a value in Go?
How to check if a value exists in a map in Golang
- Traverse through the entire map.
- Check if the present value is equal to the value the user wants to check. If it is equal and is found, print the value. Otherwise, print that the value is not found in the map.
How do you print out all keys currently stored in a map?
Using toString() For displaying all keys or values present on the map, we can simply print the string representation of keySet() and values() , respectively. That’s all about printing out all keys and values from a Map in Java.
How do you check if a map contains a key in go?
Checking if a Key Exists in a Map in Go
-
- import “fmt”
-
- func main() {
-
- mymap := make(map[string]int)
-
Can Map store duplicate values?
Map does not supports duplicate keys. you can use collection as value against same key. Because if the map previously contained a mapping for the key, the old value is replaced by the specified value.
How do you get a map key?
Java Program to Get key from HashMap using the value
- entry.getValue() – get value from the entry.
- entry.getKey() – get key from the entry.
How do I store references in a map?
You can’t store references in Standard Library containers – your map should look like: map mymap; The map will manage both the key string and the struct instances, which will be copies, for you. Both map and unordered_map work in the same way in this regard, as do all other Standard Library containers.
What is the use of map find in C++?
The map::find() is a built-in function in C++ STL which returns an iterator or a constant iterator that refers to the position where the key is present in the map. If the key is not present in the map container, it returns an iterator or a constant iterator which refers to map.end() .
What is the return value of map?
Return Value: The function returns an iterator or a constant iterator which refers to the position where the key is present in the map. If the key is not present in the map container, it returns an iterator or a constant iterator which refers to map.end (). The time complexity for searching elements in std::map is O (log n).
How do you define a reference?
You should think of a reference as a ‘const pointer to a non-const object’: Furthermore, a reference can only be built as an alias of something which exists (which is not necessary for a pointer, though advisable apart from NULL).