How do you read fstream?
Table of Contents
How do you read fstream?
In order for your program to read from the file, you must:
- include the fstream header file with using std::ifstream;
- declare a variable of type ifstream.
- open the file.
- check for an open file error.
- read from the file.
- after each read, check for end-of-file using the eof() member function.
How do I write to fstream?
In order for your program to write to a file, you must:
- include the fstream header file and using std::ostream;
- declare a variable of type ofstream.
- open the file.
- check for an open file error.
- use the file.
- close the file when access is no longer needed (optional, but a good practice)
How do I use a CPP file?
To create a file, use either the ofstream or fstream class, and specify the name of the file. To write to the file, use the insertion operator ( << ).

What is Freopen?
The freopen() function opens the new file associated with stream with the given mode, which is a character string specifying the type of access requested for the file. You can also use the freopen() function to redirect the standard stream files stdin , stdout , and stderr to files that you specify.
What is fstream in C?
In C ++ you declare variables of the ofstream and ifstream classes to get output and input file streams, respectively. Output streams are used to write to files just as you would cout. Input streams are used to read from files just as you would cin. Opening the file is done automatically by the stream’s constructor.
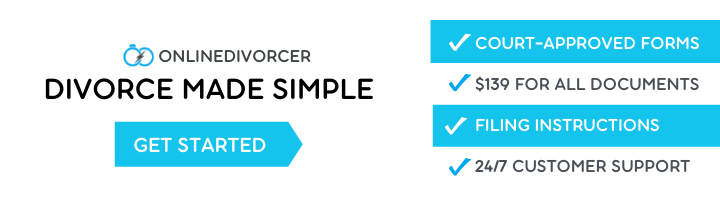
How do you read Java?
In How To Read Java: Understanding, debugging, and optimizing JVM applications you will learn how to:
- Determine what code does the first time you see it.
- Expose code logic problems.
- Evaluate heap dumps to find memory leaks.
- Monitor CPU consumption to optimize execution.
- Use thread dumps to find and solve deadlocks.
How read and write data from a file in C++?
It is used to read from files. It can perform the function of both ofstream and ifstream which means it can create files, write on files, and read from files….Opening a file.
Mode | Description |
---|---|
ios::ate | opens a file for output and move the read/write control to the end of the file. |
ios::in | opens a text file for reading. |
What is the difference between ofstream and fstream?
fstream inherits from iostream , which inherits from both istream and stream . Generally ofstream only supports output operations (i.e. textfile << “hello”), while fstream supports both output and input operations but depending on the flags given when opening the file.
What is Fmemopen?
Description. The fmemopen subroutine associates the buffer given by the buf and size arguments with a stream. The buf argument must be either a null pointer or point to a buffer that contains the value specified by the size parameter in bytes.
What is the use of Freopen?
The freopen function first attempts to close the file opened using stream . After the file is closed, it attempts to open the filename specified by the argument filename (if it is not null) in the mode specified by the argument mode . Finally it associates the file with the file stream stream .