How do you parse arguments in Python?
Table of Contents
How do you parse arguments in Python?
Argument Parsing using sys. Your program will accept an arbitrary number of arguments passed from the command-line (or terminal) while getting executed. The program will print out the arguments that were passed and the total number of arguments. Notice that the first argument is always the name of the Python file.
What is Python Argparse?
argparse is the “recommended command-line parsing module in the Python standard library.” It’s what you use to get command line arguments into your program.
How do you add an argument in Python?
To add arguments to Python scripts, you will have to use a built-in module named “argparse”. As the name suggests, it parses command line arguments used while launching a Python script or application. These parsed arguments are also checked by the “argparse” module to ensure that they are of proper “type”.
How do you handle a command line argument in Python?

To use getopt module, it is required to remove the first element from the list of command-line arguments.
- Syntax: getopt.getopt(args, options, [long_options])
- Parameters:
- args: List of arguments to be passed.
- options: String of option letters that the script want to recognize.
What are arguments in Python?
The terms parameter and argument can be used for the same thing: information that are passed into a function. From a function’s perspective: A parameter is the variable listed inside the parentheses in the function definition. An argument is the value that are sent to the function when it is called.
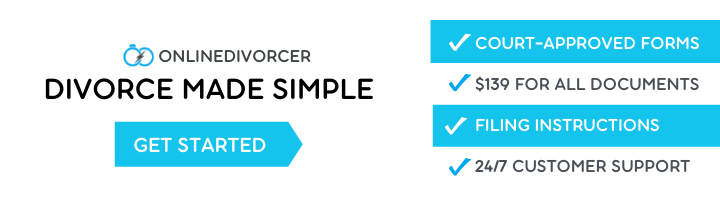
How does argument parser work?
The standard Python library argparse used to incorporate the parsing of command line arguments. Instead of having to manually set variables inside of the code, argparse can be used to add flexibility and reusability to your code by allowing user input values to be parsed and utilized.
What is positional argument in Python?
A positional argument in Python is an argument whose position matters in a function call. You have likely used positional arguments without noticing. For instance: def info(name, age): print(f”Hi, my name is {name}.
How do you handle command line arguments?
To pass command line arguments, we typically define main() with two arguments : first argument is the number of command line arguments and second is list of command-line arguments. The value of argc should be non negative. argv(ARGument Vector) is array of character pointers listing all the arguments.
How do you pass a file as an argument in Python?
“pass a file as argument in python sys argv” Code Answer
- import sys.
- print(“This is the name of the script:”, sys. argv[0])
- print(“Number of arguments:”, len(sys. argv))
- print(“The arguments are:” , str(sys. argv))
-
- #Example output.
- #This is the name of the script: sysargv.py.
- #Number of arguments in: 3.
What are the 3 types of arguments in Python?
Hence, we conclude that Python Function Arguments and its three types of arguments to functions. These are- default, keyword, and arbitrary arguments.
What is positional and keyword argument?
A positional argument means its position matters in a function call. A keyword argument is a function argument with a name label. Passing arguments as keyword arguments means order does not matter.
What is command line arguments in Python?
Python Command line arguments are input parameters passed to the script when executing them. Almost all programming language provide support for command line arguments. Then we also have command line options to set some specific options for the program.
Why do we use command line arguments?
Command-line arguments are useful when you want to control your program from outside rather than hard coding the values inside the code. To allow the usage of standard input and output so that we can utilize the shell to chain commands. To override defaults and have more direct control over the application.