How do you implement Observer design pattern?
Table of Contents
How do you implement Observer design pattern?
Design Patterns – Observer Pattern
- Create Subject class. Subject.java import java.
- Create Observer class. Observer.java public abstract class Observer { protected Subject subject; public abstract void update(); }
- Create concrete observer classes.
- Use Subject and concrete observer objects.
- Verify the output.
What is Observer design pattern in Java?
What Is the Observer Pattern? Observer is a behavioral design pattern. It specifies communication between objects: observable and observers. An observable is an object which notifies observers about the changes in its state. For example, a news agency can notify channels when it receives news.
Which interface do we need to implement the use of Observer in Java?
Here Observer and Subject are interfaces(can be any abstract super type not necessarily java interface). All observers who need the data need to implement observer interface. notify() method in observer interface defines the action to be taken when the subject provides it data.

How does the observer pattern work?
The observer pattern is a software design pattern in which an object, named the subject, maintains a list of its dependents, called observers, and notifies them automatically of any state changes, usually by calling one of their methods.
How does the observer pattern implement the Open Closed Principle?
The observer pattern allows for the Open Closed principle. This principle states that a class should be open for extensions without the need to change the class. Using the observer pattern a subject can register an unlimited number of observers.
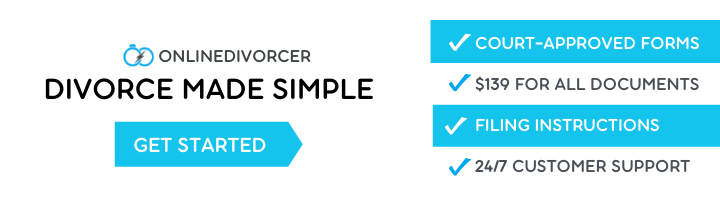
How do you implement a prototype design pattern in Java?
Design Patterns – Prototype Pattern
- Create an abstract class implementing Clonable interface.
- Create concrete classes extending the above class.
- Create a class to get concrete classes from database and store them in a Hashtable.
- PrototypePatternDemo uses ShapeCache class to get clones of shapes stored in a Hashtable.
How do observers work Java?
Behavior. In Java Edition, an observer detects changes in its target’s block states, or the breaking or placing of a block (i.e. changes in its block state, but not its block entity data). This means that changes like the age of crops can be detected because they are part of the block states.
Which pattern should be used for file system implementation?
File System implementations use the composite design pattern as described previously.
How do observers work in Java?
Observer is a behavioral design pattern that allows some objects to notify other objects about changes in their state. The Observer pattern provides a way to subscribe and unsubscribe to and from these events for any object that implements a subscriber interface.
How are Observer and observable used Java?
The Java language supports the MVC architecture with two classes: Observer : Any object that wishes to be notified when the state of another object changes. Observable : Any object whose state may be of interest, and in whom another object may register an interest.
What are two strategies to implement OCP?
These five principles are:
- The Single Responsibility Principle: A class should have one, and only one, reason to change.
- The Open Closed Principle: Software entities should be open to extension but closed to modification.
- The Liskov Substitution Principle: Derived classes must be substitutable for their base classes.
Is Observer pattern event driven?
The Observer design pattern is modeled on the event-driven programming paradigm . Just like other design patterns, this pattern lets us define loosely coupled systems. Leveraging this pattern, we can write maintainable and modular software.
What operations is essential to implement the prototype design pattern?
Prototype patterns are required, when object creation is time consuming, and costly operation, so we create objects with the existing object itself. One of the best available ways to create an object from existing objects is the clone() method. Clone is the simplest approach to implement a prototype pattern.
Which of the following classes is an implementation of template method pattern?
The Abstract Class declares methods that act as steps of an algorithm, as well as the actual template method which calls these methods in a specific order. The steps may either be declared abstract or have some default implementation. Concrete Classes can override all of the steps, but not the template method itself.
How do you implement a file system?
To grow a file:
- Load the file’s associated inode into memory.
- Allocate disk blocks (mark them as allocated in your freelist).
- Modify the file’s inode to point to these blocks.
- Write the data the user gives to these blocks.
- Flush all modifications to disk.
How many design patterns are there in Java?
23 design patterns
As per the design pattern reference book Design Patterns – Elements of Reusable Object-Oriented Software , there are 23 design patterns which can be classified in three categories: Creational, Structural and Behavioral patterns.
What is difference between Observer and Observable?
Observer : Any object that wishes to be notified when the state of another object changes. Observable : Any object whose state may be of interest, and in whom another object may register an interest.