How do you divide code in Java?
Table of Contents
How do you divide code in Java?
Examples of Division Use in Java
- int result = 10 / 5;
- // statement 1.
- int result = 11 / 5;
- // statement 2.
- double result = 10.0 / 5.0;
- // statement 3.
- double result = 11.0 / 5.0;
- // statement 4.
Does Java have division?
Java does integer division, which basically is the same as regular real division, but you throw away the remainder (or fraction). Thus, 7 / 3 is 2 with a remainder of 1. Throw away the remainder, and the result is 2. Integer division can come in very handy.
How do you write division codes?

- int divide(int x, int y) { // handle divisibility by 0. if (y == 0)
- printf(“Error!! Divisible by 0”); exit(-1); } // store sign of the result.
- if (x * y < 0) { sign = -1; } return sign * division(abs(x), abs(y));
- } int main(void) {
- int divisor = -7; printf(“The quotient is %d\n”, divide(dividend, divisor)); return 0;
How do you multiply and divide in Java?
Of course Java can also do multiplication and division. Since most keyboards don’t have the times and division symbols you learned in grammar school, Java uses * to mean multiplication and / to mean division.
What is floor division in Java?
floorDiv() is used to find the largest integer value that is less than or equal to the algebraic quotient. This method first divide the first argument by the second argument and then performs a floor() operation over the result and returns the integer that is less or equal to the quotient.
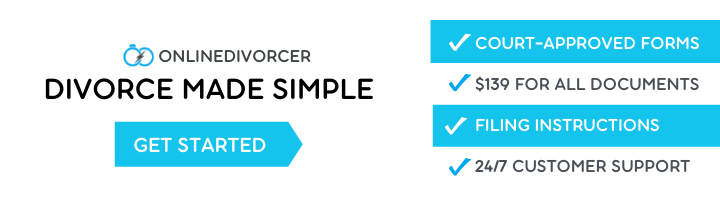
How do you divide decimals in Java?
If you need to divide two integers and get a decimal result, you can cast either the numerator or denominator to double before the operation. X Research source is performed. In this example, we’ll cast a to double so we get a decimal result: int a = 55; int b = 25; double r = (double) a / b // The answer is 2.2.
How do you divide a remainder in Java?
The % operator returns the remainder of two numbers. For instance 10 % 3 is 1 because 10 divided by 3 leaves a remainder of 1. You can use % just as you might use any other more common operator like + or – . Perhaps surprisingly the remainder operator can be used with floating point values as well.
How do you write Math equations in Java?
Java Math
- Math.max(x,y) The Math.max(x,y) method can be used to find the highest value of x and y:
- Math.min(x,y) The Math.min(x,y) method can be used to find the lowest value of x and y:
- Math.sqrt(x) The Math.sqrt(x) method returns the square root of x:
- Math.abs(x)
How do you float a division in Java?
float v=s/t performs division then transforms result into a float. float v=(float)s/t casts to float then performs division.
How do you divide precision in Java?
BigDecimal. divide(BigDecimal divisor, int scale, RoundingMode roundingMode) returns a BigDecimal whose value is (this / divisor), and whose scale is as specified. If rounding must be performed to generate a result with the specified scale, the specified rounding mode is applied.
What is a div operator?
The division operator ( / ) produces the quotient of its operands where the left operand is the dividend and the right operand is the divisor.
What does div stand for in coding?
: The Content Division element.
How do you write a quotient in Java?
Using Math.DivRem() method
- using System;
- public class JTP {
- public static void Main(){
- int dividend = 34, divisor = 3, remainder = 0;
- int quotient = Math.DivRem(dividend, divisor, out remainder);
- Console.WriteLine(“Quotient = ” + quotient);
- Console.WriteLine(“Remainder = ” + remainder);
- }
What is Math function Java?
The java. lang. Math class contains methods for performing basic numeric operations such as the elementary exponential, logarithm, square root, and trigonometric functions. This class provides mathematical functions in Java.
How do you call a Math class in Java?
Syntax
- import java.lang.Math;
- public class MathClassExample5 {
- public static void main(String[] args) {
- double x = 45;
- double y = -180;
- x = Math.toRadians(x);
- y = Math.toRadians(y);
- System.out.println(“Math.toRadius() of x = ” + Math.toRadians(x));