How do you create a list of strings and integers in Java?
Table of Contents
How do you create a list of strings and integers in Java?
Using Arrays. asList()
- Creating Immutable List. Arrays. asList() creates an immutable list from an array. Hence it can be used to instantiate a list with an array.
- Creating Mutable List. Syntax: List list=new ArrayList<>(Arrays.asList(1, 2, 3)); Examples: import java.util.ArrayList;
Can we add string and int in list Java?
No it isn’t, unless you have a List And even then you’d need to . add(new Integer(a1)) since int is not a class.
Can we store string and integer together in list?

Sure. You can put any members in an object you like. For example, this class stores a string and 11 integers. The integers are stored in an array.
How do you store a string and integer together in an ArrayList?
For example, to add elements to the ArrayList , use the add() method:
- import java. util.
- public class Main { public static void main(String[] args) { ArrayList cars = new ArrayList(); cars. add(“Volvo”); cars.
- Create an ArrayList to store numbers (add elements of type Integer ): import java. util.
How do I make a list of strings in Java?
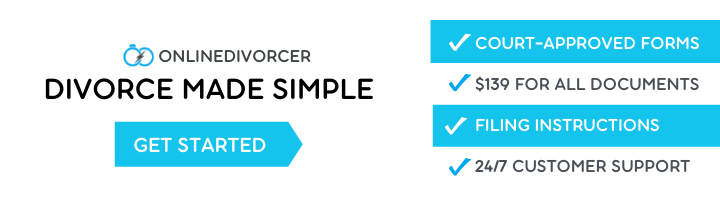
We can create List as: List arrayList = new ArrayList<>(); List linkedList = new LinkedList<>(); We can also create a fixed-size list as: List list = Arrays.
How do you add integers to a list in Java?
Using Stream.collect() The second method for calculating the sum of a list of integers is by using the collect() terminal operation: List integers = Arrays. asList(1, 2, 3, 4, 5); Integer sum = integers. stream() .
Can we add integer and string in ArrayList in Java?
Yeah, you can create an ArrayList but having said that, my advice for you is this: don’t. Don’t create Lists with mixed types since this suggests that your program design is broken and needs to be improved so that this sort of monster isn’t needed.
Can we concat string and integer?
To concatenate a string to an int value, use the concatenation operator. Here is our int. int val = 3; Now, to concatenate a string, you need to declare a string and use the + operator.
Can we store string and integer together in an ArrayList in Java?
So, you can use ArrayList> .
How do you input an int into a list?
First, decide the size of the list. Get numbers from the user using the input() function. Split it string on whitespace and convert each number to an integer using an int() function. Add all that numbers to the list.
How do you add an integer to an ArrayList?
So, to create an ArrayList of ints, we need to use the Integer wrapper class as its type. We can now add integers to the ArrayList by using the class’s add() method. ArrayList , just like normal arrays, follow zero-based indexing. We can specify the index where we want to add an object in the add() method.
How do I make a list of strings?
To create a list of strings, first use square brackets [ and ] to create a list. Then place the list items inside the brackets separated by commas. Remember that strings must be surrounded by quotes. Also remember to use = to store the list in a variable.
How do I assign a list of strings?
You can do it this way : List dataList = new List(); int len = info. length; for (int i = 0; i < len; i++) dataList.
How do you add numbers to an ArrayList?
Adding values to Arraylist
- ArrayList arr = new ArrayList(); arr. add(3); arr. add(“ss”); Code 2:
- ArrayList arr = new ArrayList(); arr. add(3); arr. add(“ss”); Code 3:
- ArrayList arr = new ArrayList(); arr. add(new Integer(3)); arr. add(new String(“ss”));
How do I return an integer from a list in Java?
The solution to this has been provided above by the other coders: either you can change the return type of your getUnit() method to an integer array and returning the reference of that array or you can change the return type of your getUnit() method to an integer list and return the reference of the list.
How do you add a integer to a string in Java?
To concatenate a String and some integer values, you need to use the + operator. Let’s say the following is the string. String str = “Demo Text”; Now, we will concatenate integer values.
Can ArrayList store integers?
An ArrayList cannot store ints. To place ints in ArrayList, we must convert them to Integers. This can be done in the add() method on ArrayList.