How do you capitalize the first letter of a word in python?
Table of Contents
How do you capitalize the first letter of a word in python?
Use title() to capitalize the first letter of each word in a string in python. Python Str class provides a member function title() which makes each word title cased in string. It means, it converts the first character of each word to upper case and all remaining characters of word to lower case.
How will you capitalizes first letter of string?
To capitalize the first character of a string, We can use the charAt() to separate the first character and then use the toUpperCase() function to capitalize it.
How do I print the first letter of every word in python?
“print first letter of every word in the string python” Code Answer’s

- def print_first_word():
- words = “All good things come to those who wait”
- print(words. split(). pop(0))
- #to print the last word use pop(-1)
- print_first_word()
How do I change the first letter of a string in python?
Use Python built-in replace() function to replace the first character in the string. The str. replace takes 3 parameters old, new, and count (optional). Where count indicates the number of times you want to replace the old substring with the new substring.
How do you capitalize specific letters in python?
To capitalize the first letter, use the capitalize() function. This functions converts the first character to uppercase and converts the remaining characters to lowercase. It doesn’t take any parameters and returns the copy of the modified string.
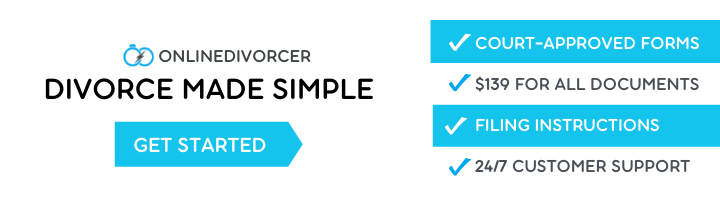
How do you capitalize the first letter of every word?
To use a keyboard shortcut to change between lowercase, UPPERCASE, and Capitalize Each Word, select the text and press SHIFT + F3 until the case you want is applied.
How do you capitalize the first and last letters of a word in Python?
2 comments on “Python program to capitalize the first and last letter of each word of a string”
- BHANU. a=input() print(a[0].upper()+a[1:len(a)-1]+a[len(a)-1].upper())
- yaminipolisetti98435. s=input() n=len(s) print(s[0].upper()+s[1:n-1]+s[-1].upper())
How do you capitalize the first and last letter in Python?
How do you capitalize a string in Python?
Python String capitalize() The capitalize() method converts the first character of a string to an uppercase letter and all other alphabets to lowercase.
How do you get the first letter of a string in a list Python?
“grab the first letter of each string in an array python” Code Answer’s
- def abbrevName(name):
- xs = (name)
- name_list = xs. split()
- # print(name_list)
-
- first = name_list[0][0]
- second = name_list[1][0]
-
How can I get the first letter of each word in a string?
To get the first letter of each word in a string:
- Call the split() method on the string to get an array containing the words in the string.
- Call the map() method to iterate over the array and return the first letter of each word.
- Join the array of first letters into a string, using the join() method.
How do I change the first letter of a string?
To replace the first character in a string: Call the replace() method passing it the variable as the first parameter and the replacement character as the second, e.g. str. replace(char, ‘a’) The replace method will return a new string with the first character replaced.
How do you change a specific letter in a string in Python?
replace() method helps to replace the occurrence of the given old character with the new character or substring. The method contains the parameters like old(a character that you wish to replace), new(a new character you would like to replace with), and count(a number of times you want to replace the character).
How do you capitalize the first and last letter of a string in Python?
How do you capitalize a letter?
Why is first letter of each word capitalized?
It’s a traditional way of capitalizing titles, it’s normally not to be used in articles (though nobody really prohibits it) – there Sentence case is the most widely used. In some regions it’s considered more academic and thus could give a higher esteem to the writer.
Why is the first letter capitalized?
Capital letters are useful signals for a reader. They have three main purposes: to let the reader know a sentence is beginning, to show important words in a title, and to signal proper names and official titles. 1. Capitals signal the start of a new sentence.
What does Startswith mean in python?
The startswith() method returns True if the string starts with the specified value, otherwise False.
How do you convert the first character of each element in a series to uppercase?
In python, if we wish to convert only the first character of every word to uppercase, we can use the capitalize() method. Or we can take just the first character of the string and change it to uppercase using the upper() method.