How do you add a variable to a string in Ruby?
Table of Contents
How do you add a variable to a string in Ruby?
Instead of terminating the string and using the + operator, you enclose the variable with the #{} syntax. This syntax tells Ruby to evaluate the expression and inject it into the string.
What is string interpolation rails?
String Interpolation, it is all about combining strings together, but not by using the + operator. String Interpolation works only when we use double quotes (“”) for the string formation. String Interpolation provides an easy way to process String literals.
How do you pass a string in Ruby?
Ruby is strictly pass-by-value, always. There is no pass-by-reference in Ruby, ever.

What is string in Ruby on Rails?
In Ruby, string is a sequence of one or more characters. It may consist of numbers, letters, or symbols. Here strings are the objects, and apart from other languages, strings are mutable, i.e. strings can be changed in place instead of creating new strings.
What is string concatenation in Ruby?
concat is a String class method in Ruby which is used to Concatenates two objects of String. If the given object is an Integer, then it is considered a codepoint and converted to a character before concatenation. Syntax:String_Object.concat(String_Object)
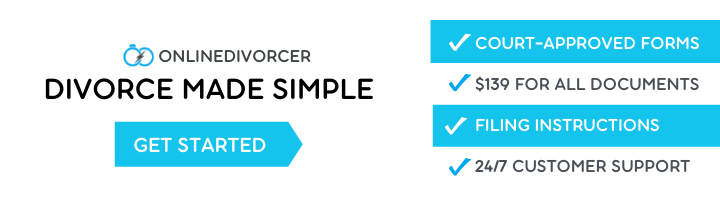
How do you convert an int to a string in Ruby?
Ruby provides the to_i and to_f methods to convert strings to numbers. to_i converts a string to an integer, and to_f converts a string to a float.
How do I concatenate strings in Ruby on Rails?
You can use the + operator to append a string to another. In this case, a + b + c , creates a new string. Btw, you don’t need to use variables to make this work.
How do you join two strings in Ruby?
concat is a String class method in Ruby which is used to Concatenates two objects of String. If the given object is an Integer, then it is considered a codepoint and converted to a character before concatenation. Parameters: This method can take the string object and normal string as the parameters.
What are the string methods in Ruby?
17 Useful Ruby String Methods to Clean and Format Your Data
- Iterate over each character of a String.
- Convert a String to a character array.
- Get the length of a String.
- Get the count of characters of a String.
- Reverse a String.
- 6 Search for one or more characters of a String.
- Replace characters in a String.
- Split a String.
How do you access a character in a string in Ruby?
Accessing Characters Within a String You can also access a single character from the end of the string with a negative index. -1 would let you access the last character of the string, -2 would access the second-to-last, and so on. This can be useful for manipulating or transforming the characters in the string.
How do I convert a string to an array in Ruby?
The general syntax for using the split method is string. split() . The place at which to split the string is specified as an argument to the method. The split substrings will be returned together in an array.
How do you join a string array in Ruby?
In Ruby, we use #concat to append a string to another string or an element to the array.
How do I use a variable in Ruby?
Variable Naming Conventions in Ruby
- You can only create the name from alphanumeric characters or an underscore.
- The name of a variable cannot begin with a numerical value.
- Names in Ruby are case-sensitive.
- Variable names cannot begin in an Uppercase letter.
- Variable names cannot contain special characters.
How do I find a string in Ruby?
include? is a String class method in Ruby which is used to return true if the given string contains the given string or character.
- Syntax: str. include?
- Parameters: Here, str is the given string.
- Returns: true if the given string contains the given string or character otherwise false.
What is a string variable in Java?
Variables are containers for storing data values. In Java, there are different types of variables, for example: String – stores text, such as “Hello”. String values are surrounded by double quotes. int – stores integers (whole numbers), without decimals, such as 123 or -123.