How do I calculate the number of days between two dates in C#?
Table of Contents
How do I calculate the number of days between two dates in C#?
The difference between two dates can be calculated in C# by using the substraction operator – or the DateTime. Subtract() method.
How do you find the difference between two dates in C sharp?
How to find date difference in C#
- DateTime newDate = new DateTime(2000, 5, 1); Here newDate represents year as 2000 and month as May and date as 1 .
- System.TimeSpan diff = secondDate.Subtract(firstDate);
- String diff2 = (secondDate – firstDate).TotalDays.ToString();
How do I get the difference between two dates and minutes in C#?
DateTime date1 = new DateTime(2018, 7, 15, 08, 15, 20); DateTime date2 = new DateTime(2018, 8, 17, 11, 14, 25); Now, calculate the difference between two dates. TimeSpan ts = date2 – date1; To calculate minutes.
What is SQL datediff?
DATEDIFF() in SQL It returns the number of times it crossed the defined date part boundaries between the start and end dates (as a signed integer value).
How do you find the difference between two dates and minutes?
JavaScript Datetime: Exercise-44 with Solution
- Sample Solution:-
- HTML Code:
- JavaScript Code: function diff_minutes(dt2, dt1) { var diff =(dt2.getTime() – dt1.getTime()) / 1000; diff /= 60; return Math.abs(Math.round(diff)); } dt1 = new Date(2014,10,2); dt2 = new Date(2014,10,3); console.log(diff_minutes(dt1, dt2));
How do I find the number of days between two dates in SQL?
The DATEDIFF() function returns the difference between two dates.
How can I calculate days between two dates in SQL?
The statement DATEDIFF(dd,@fromdate,@todate) + 1 gives the number of dates between the two dates. The statement DATEDIFF(wk,@fromdate,@todate) gives the number of weeks between dates and * 2 gives us the weekend (Saturday and Sunday) count. The next two statements excludes the day if it’s a Saturday or Sunday.
How do I get the number of days between two dates in SQL?
Use the DATEDIFF() function to retrieve the number of days between two dates in a MySQL database. This function takes two arguments: The end date. (In our example, it’s the expiration_date column.)

How do I add TimeSpan to DateTime?
Add DateTime and TimeSpan in C#.Net
- using System;
- public class MainClass{
- public static void Main(String[] argv){
- DateTime today = DateTime.Now;
- TimeSpan duration = new TimeSpan(36, 0, 0, 0);
- DateTime answer = today.Add(duration);
- Console.WriteLine(“{0:dddd}”, answer);
- }
How do you declare a TimeSpan?
The following example initializes a TimeSpan value to a specified number of hours, minutes, and seconds. TimeSpan interval = new TimeSpan(2, 14, 18); Console. WriteLine(interval. ToString()); // Displays “02:14:18”.
How do you check if a date is before another date C#?
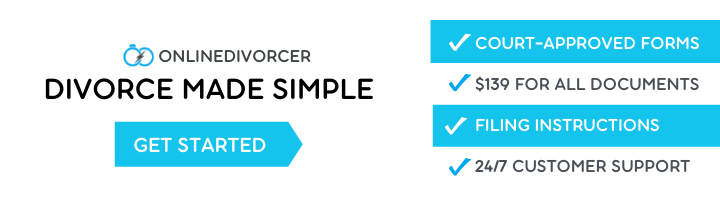
Compare() Method in C# This method is used to compare two instances of DateTime and returns an integer that indicates whether the first instance is earlier than, the same as, or later than the second instance.
How can I get minutes between two dates in SQL?
To calculate the difference between the arrival and the departure in T-SQL, use the DATEDIFF(datepart, startdate, enddate) function. The datepart argument can be microsecond , second , minute , hour , day , week , month , quarter , or year .
How do you find the difference between two date hours?
To calculate the hours difference of the two dates, we should divide the diffInMilliSeconds with 3600 which is the milliseconds of 1 hour, and then further divide it by 24 (24 hours per day) and get the remainder using modules operator.