Can you loop through an ArrayList?
Table of Contents
Can you loop through an ArrayList?
An Iterator can be used to loop through an ArrayList. The method hasNext( ) returns true if there are more elements in ArrayList and false otherwise.
What is the simplest way of looping through an array?
- . forEach() loop: Each element of the array is subjected to a function performing some operation or task.
- for…of Loop: This is a new version of for loop. This loop requires assigning a temporary name to each of the element of the array.
- Comparison of performance of loops available in JavaScript :
How do you make a for loop run infinitely?
To make an infinite loop, just use true as your condition. true is always true, so the loop will repeat forever.
How do you iterate through values in an array?

How to Loop through an Array in JavaScript
- Initialization – initializes the loop variable with a starting value which can only be executed once.
- Condition – specifies that the loop should stop looping.
- Final expression – is performed at the end of each loop execution. It is used to increment the index.
What is the fastest way to loop through an array in JavaScript?
- The fastest loop is a for loop, both with and without caching length delivering really similar performance.
- The while loop with decrements was approximately 1.5 times slower than the for loop.
- A loop using a callback function (like the standard forEach), was approximately 10 times slower than the for loop.
What is the safest way to loop through an array in JavaScript?
forEach(callback) method is an efficient way to iterate over all array items. Its first argument is the callback function, which is invoked for every item in the array with 3 arguments: item, index, and the array itself.
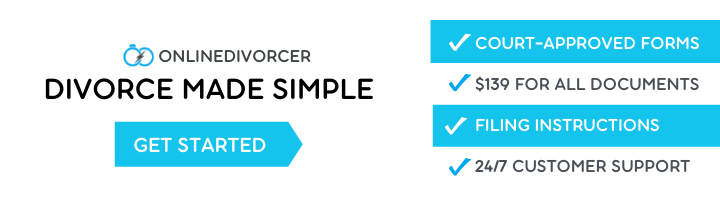
How do you run an infinite loop in Javascript?
In for() loop: To avoid ending up in an infinite loop while using a for statement, ensure that the statements in the for() block never change the value of the loop counter variable. If they do, then your loop may either terminate prematurely or it may end up in an infinite loop.
Can for loop go infinite?
A for loop is only another syntax for a while loop. Everything which is possible with one of them is also possible with the other one. Any for loop where the termination condition can never be met will be infinite: for($i = 0; $i > -1; $i++) { }
How will you loop through this array in JavaScript?
- There are multiple ways one can iterate over an array in Javascript. The most useful ones are mentioned below.
- Using while loop. This is again similar to other languages.
- using forEach method.
- Using every method.
- Using map.
- Using Filter.
- Using Reduce.
- Using Some.
Can an enhanced for loop modify an array?
Bookmark this question. Show activity on this post. When would this actually affect array A? We learned in class that an Enhanced For loops doesn’t actually modify the underlying array but that there some special cases.
Which is the most preferred loop structure to iterate an array?
Using for-each Loop However, the for-each loop is easier to write and understand. This is why the for-each loop is preferred over the for loop when working with arrays and collections.
What is infinite loop give example?
What is an Infinite Loop? An infinite loop occurs when a condition always evaluates to true. Usually, this is an error. For example, you might have a loop that decrements until it reaches 0.