Can we use == to compare two float values in Python?
Table of Contents
Can we use == to compare two float values in Python?
In the case of floating-point numbers, the relational operator (==) does not produce correct output, this is due to the internal precision errors in rounding up floating-point numbers. In the above example, we can see the inaccuracy in comparing two floating-point numbers using “==” operator.
Can we compare integer with float?
So as long your floats come from non decimal literals, the comparison with the equivalent integer will be the same, because the integer will be cast to the very same float before the comparison can be done.
Can we compare int and float in Python?
In Python, 3 is int and 3.0 is float. Both types are different but both has the same value, that is 3 == 3.0 is true. if 3 == 3.0: print(‘3 is 3.0.

How do you compare two floating point numbers?
To compare two floating point values, we have to consider the precision in to the comparison. For example, if two numbers are 3.1428 and 3.1415, then they are same up to the precision 0.01, but after that, like 0.001 they are not same.
How do you compare two decimal numbers in Python?
Decimal#compare() : compare() is a Decimal class method which compares the two Decimal values.
- Syntax: Decimal.compare()
- Parameter: Decimal values.
- Return: 1 – if a > b. -1 – if a < b. 0 – if a = b.
How do you get 2 numbers after the decimal point in Python?
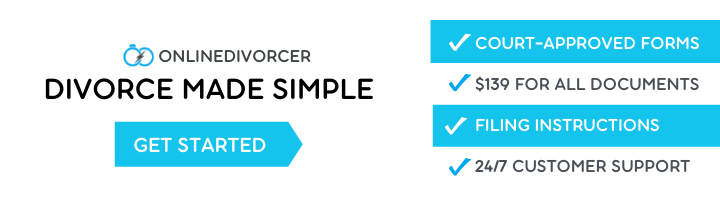
Use str. format() with “{:. 2f}” as string and float as a number to display 2 decimal places in Python. Call print and it will display the float with 2 decimal places in the console.
Is float equal to double?
Float and double Double is more precise than float and can store 64 bits, double of the number of bits float can store. Double is more precise and for storing large numbers, we prefer double over float. For example, to store the annual salary of the CEO of a company, double will be a more accurate choice.
Is 3.0 and 3 the same in Python?
Here, 3 is an int, and 3.0 is a float, but 3 isn’t lesser than 3.0, or vice versa. This one results in True because when comparing strings, their ASCII values are compared. The ASCII value for ‘A’ is 65, but that for ‘a’ is 97.
Does == work for floats?
Because floating point arithmetic is different from real number arithmetic. Bottom line: Never use == to compare two floating point numbers. Here’s a simple example: double x = 1.0 / 10.0; double y = x * 10.0; if (y !=
How do you compare two decimal numbers?
The rules for comparing decimal numbers is as follows:
- Compare the whole number part first (i.e., compare the digits in front of the decimal). If they are different, then compare the numbers as you would for any whole number.
- Compare the tenths place.
- Compare the hundredths place.
- Etc.
How do you round a float to two decimal places in Python?
Python’s round() function requires two arguments. First is the number to be rounded. Second argument decides the number of decimal places to which it is rounded. To round the number to 2 decimals, give second argument as 2.
Is 1.1 a float or double?
Now float can take only some digits after the decimal place whereas double can take larger number of digits after the decimal place. Thus the value of 1.1 in float is different from the value of 1.1 stored in double.
Is 99.9 double or float?
double
Floating-point numbers are by default of type double. Therefore 99.9 is a double, not a float. What is a double data type example? Double can store numbers between the range -1.7 x 10308 to +1.7 x 10308.
How do you compare numbers in Python?
Python comparison operators can be used to compare strings in Python. These operators are: equal to ( == ), not equal to ( != ), greater than ( > ), less than ( < ), less than or equal to ( <= ), and greater than or equal to ( >= ).
Is int 3 and float 3.0 are equal?
The integer 3 and the floating-point number 3.0 clearly have the same value, so if(3 == 3.0) is true.
How do you find a floating point number in Python?
Use isinstance() to check if a number is an int or float
- number = 25.9.
- check_int = isinstance(25.9, int)
- print(check_int)
- check_float = isinstance(25.9, float)
- print(check_float)
How did you compare decimal number in a?
Decimal numbers are compared in the same way as other numbers: by comparing the different place values from left to right. We use the symbols <, > and = to compare decimals as shown below. When comparing two decimals, it is helpful to write one below the other.
How do you compare decimals examples?
When we need to compare decimals and fractions, we first convert the given fraction to a decimal number and then compare them using the same procedure. For example, let us compare 0.528 and 3/7. In this case, we will convert 3/7 to a decimal number by dividing 3 by 7. So, 3 ÷ 7 = 0.428.